Before we get around to explaining how to create software using Python, let’s list down some basic differences between an actual software and the small scripts.
- A Graphical User Interface (GUI)
- Exception Handling
- Logging System
- Software is compiled code that is executed
- Scripts are interpreted
- Script are typically a single file program
- Software may consists of several smaller programs working together
Note, this article only covers the theory of creating software in Python and the problems associated with it. It is not a magic way to create software for someone with no or little prior experience in Python. While this article is open for all, only people who have cemented their fundamental concepts in Python should actually attempt to create actual software. You can learn these skills at our site, CodersLegacy.
Required Python Skills
It is fairly obvious that anyone attempting to create software should be sufficiently skilled in the core concepts of Python. However we’ll be discussing certain types of skills here that aren’t part of the core concepts (loops, functions, File Handling), but essential when it comes to making software. You can skim over this section if you read our Top Coding Practices article instead. Everything is explained in much more detail there with supporting code and images.
There are several things that are acceptable while you are simply creating scripts or practicing programming, such as taking input from the console, debugging with the use of print statements, letting errors crash your program etc. However, when making software, especially software made for distribution to users, several things must be kept in mind.
Three main things which we’ll discuss briefly here are Logging, GUI’s and Exception handling. Have you every used a software without a helpful User interface? Would you pick a console based software over a GUI based software? For obvious reasons, all software should have a helpful and user friendly GUI. If you don’t know how to implement a GUI, head over to our Python GUI section where the various GUI libraries are discussed (After you read this article that is).
Next up is Exception handling. When an error occurs, your entire program crashes. This is unacceptable in a software, especially larger ones. Many of us encounter errors while using software, but instead of the program crashing, an error message is displayed instead. This is exception handling, where a good implementation can catch any exceptions and take an alternate route instead to maintain stability.
Using print statements to help you during debugging is a practice discouraged at higher levels. We have a proper system for this purpose called Logging. It involves system events that occur to be saved within a file, complete with details about that event such as the exact time is occurred. This is also useful because you can ask your customers to send you their log file if their software produces an error. Using this file you can then figure out what went wrong. Learn more about logging in Python here.
Testing
At this point, we’re assuming you’ve done most of the programming using the guidelines mentioned above and are now ready for the next phase.
If you want to create software in Python, you’ll have to spend alot time in debugging and testing phases. Debugging is typically done both during and after code development. The testing phases begin only after the code is (mostly) complete though.
First up is what we call Alpha testing or “in-house” testing. This means testing done by the programmer(s) who developed the software. Next up is the Beta Testing phase, were people outside the development team are asked to test the software. Beta testing is typically done by non-programmers. From personal experience, having non-programmers do testing is very insightful. They tend to have a different mindset when approaching the software, and help expose bugs a regular programmer might not see.
Remember to test your software on a wide variety of devices and Operating systems. Rarely are two devices the same due to the increasing complexity of modern day computers. Also refrain from using Operating system specific libraries or device specific code (If you’re aiming for mobiles as well) to maintain portability across platforms. Any third party tools you include should work across all platforms you are aiming for.
Software Distribution Issues
So you’ve been able to create your Python software. You’ve completed your code, debugged and documented it. You’ve completed your own testing and maybe even had a few people test it out for you. So what’s the next step? If your software was meant for personal usage, congratulations, you’re done. But say you wanted to actually distribute your software, how would you do so? Would you simply hand out the code you’ve worked so hard to make? If so, what’s stopping people from simply taking your source code and begin distributing the software as though it were their own.
Piracy is an issue that has no definite solution. No matter how hard you try, if someone tries hard enough, your code will be cracked eventually. There are people whose entire jobs are based off preventing piracy. What you can do however, is stop the average rookie from cracking your code overnight.
Now that we’ve established that we cannot go around giving out the source code, we need to way to distribute software without the source code. This is where the concept of compiled software comes in. Compilers translate source code from a high-level programming language to a lower level language to create an executable program. This compiled program can then be distributed safely as it’s not the source code. Another benefit of compiled programs is that they tend to run faster than normal interpreted programs.
Pyinstaller
Python doesn’t natively come with a way to create compiled programs. However, pyinstaller is a python library that can be used for this purpose. You install it as you would a regular library using the pip install pyinstaller
command in the cmd, and then follow the below steps. Check our getting started with Python guide if you’re having trouble installing the library.
Go to the command prompt, and navigate to the file you wish to convert. In this case, we’ll be converting a file called “TestFile.py“. First we navigate to the directory where our File is stored. In our case, Desktop.

Once you’ve successfully reached your python file, run the above command, and pyinstaller will take care of the rest.
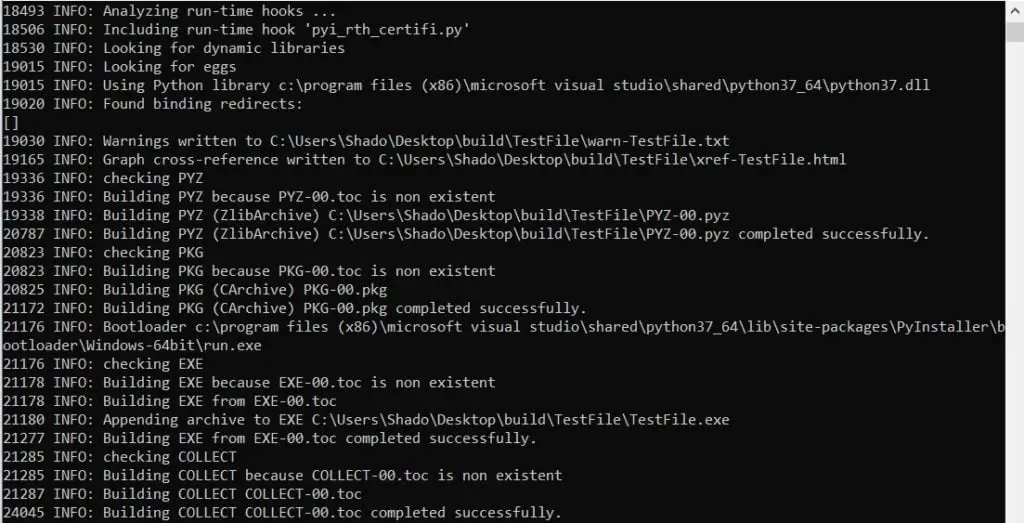
This is a very basic implementation of pyinstaller. In a more real life scenario, there will likely be many more issues. There’s a good chance the default pyinstaller compiler settings won’t work for you. Luckily there are many alternate settings available. See them below.
The –onefile setting creates a one-file bundled executable. Its a good alternative if the above code fails. By Default the –dir is picked.

Some people might want to use the –noconsole setting as it prevents any console windows from showing up. You’ll probably want this if you’re using a GUI software. Alternatively there is also the –console setting. Format is the same as the –onefile example above.
As you can see pyinstaller is a complicated beast, and is deserving of it’s own separate page, where we have discussed its settings and inner workings in greater detail.
Software Protection
One final thing we’ll be discussing here, is software protection. It is in-fact possible to convert compiled code back into source code, contrary to common belief. It’s not easy of course. And likely only possible by those with the right tools and experience, but it can be done. If you’re software is freeware or something of the sort, it shouldn’t be much of an issue. But for commercial sellers, this is a very real problem.
So what can you do to stop it? This isn’t a software security guide, so we won’t be explaining the exact code or method. Rather we’ll go through common ways and the concepts required to carry them out.
Online verification.
This method involves a registration key that your client will be given when he buys the software. When the client logins to the software, there is a check to see if the key is valid. An online request is sent out to a hosted server you have, where there is a file containing the list of valid registration keys. The registration key is checked, and a message sent back stating whether it was valid or not. In the case of an invalid key, the software will not begin. (Of course this was just my basic theory, you can come up with your techniques)
Now this may seem like a little advanced, but it’s simpler that it looks. The only hard part is getting a place to host your file. If you have a website for your software, you can simply upload the file there. Or you’ll have to get separate hosting. You could explore free hosting options too.
If you’ve gotten this far, it’s a simple matter of using python requests and some file handling to get through the rest. We’ll be going through some simpler options next.
Code Obfuscators.
Code obfuscators are accept python source code as input and produce “transformed” code which is harder to understand. The process involves removing comments, renaming variables and identifiers to gibberish and etc. This increases the effort required for some one to reverse engineer your code. You can search online for popular code obfuscators.
Timed software.
Sometimes you might be in a situation where your customer purchases your software for a limited time period. And naturally, you’re right to be concerned that he might distribute it to others. One way to handle this is cause the software to automatically deactivate once a certain date has been reached. All this requires is a 4 – 5 line script that runs as soon as the exe is clicked and checks for the date. What happens after this is up-to you. You could keep it in this state for ever, or you could cause it to self – delete itself, preventing anyone from trying to work around it.
Services
This can’t really be called a solution, but it’s something you should consider. Instead of distributing software, offer your clients your services instead. Let’s take Google for instance. Google Search Engine is a service offered by Google, not a software. Since the code was never distributed, there is no chance of anyone pirating it illegally. Same concept is applied in online games, which require online verification and are played on the servers of the company who own it.
If you can come up with a online service you can offer your clients, you won’t have to worry about protecting your software form pirates. Your objective of “Create Python software” won’t really change, but the way you deliver to your customers will change.
Updates
Once again, a rather roundabout way to prevent piracy is frequency updating with new features. If say, your software is being cracked and wide spread distributed illegally every 2-3 weeks, you can push out a new feature update every 2-3 weeks as well. People will be less inclined to use pirated versions if there is a better version out there especially if you’re software if free).
Conclusion
Some closing tips. If you’ve successfully completed all the above steps and now have your own customer base, a new set of issues arise. You’ll need to focus on marketing your software for instance. This is where social media can help you, or google ads as a paid alternative.
Secondly customer complaints will now be a pressing concern. You’ll likely have to spend a long time fixing new bugs and increasing portability across devices for your customers. This is where those log files we discussed earlier will come in handy.
Lastly, don’t forget to keep updating. With the passage of time, newer vulnerabilities come up and most importantly customers won’t trust software that hasn’t been updated in years.
This marks the end of the Create software in Python article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions can be asked in the comments section below.