Pygame is a popular library in Python for creating games and graphical applications. One of the essential tasks in creating games is loading images into the game window. Pygame provides several functions to load images from files, but what if you want to load an image from using a web image url? In this article, we will discuss how to load images from web URLs in Pygame.
Load image from Web URL in Pygame
To load an image from a web URL, we first need to download the image from the URL and then load it using Pygame’s image loading functions. We can use the requests
library in Python to download the image from the web.
Here is an example code to download and load an image from a web URL using Pygame:
import pygame
import requests
import sys
from io import BytesIO
pygame.init()
screen = pygame.display.set_mode((800, 600))
response = requests.get('https://www.denofgeek.com/wp-content/uploads/2022/05/Leged-of-Zelda-Link.jpg')
img = pygame.image.load(BytesIO(response.content))
while True: # Game Loop
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.blit(img, (0, 0))
pygame.display.update()
In this example, we first initialize Pygame and create a Pygame display surface. We then specify the web URL from where we want to download the image. We use the requests
library to download the image from the URL, which returns the image content as a bytes object. Then, we will create a Pygame image object from the bytes object using the BytesIO
class. Within the game loop, we then draw this image as our background.
But there is currently a small problem. Here is the current output.
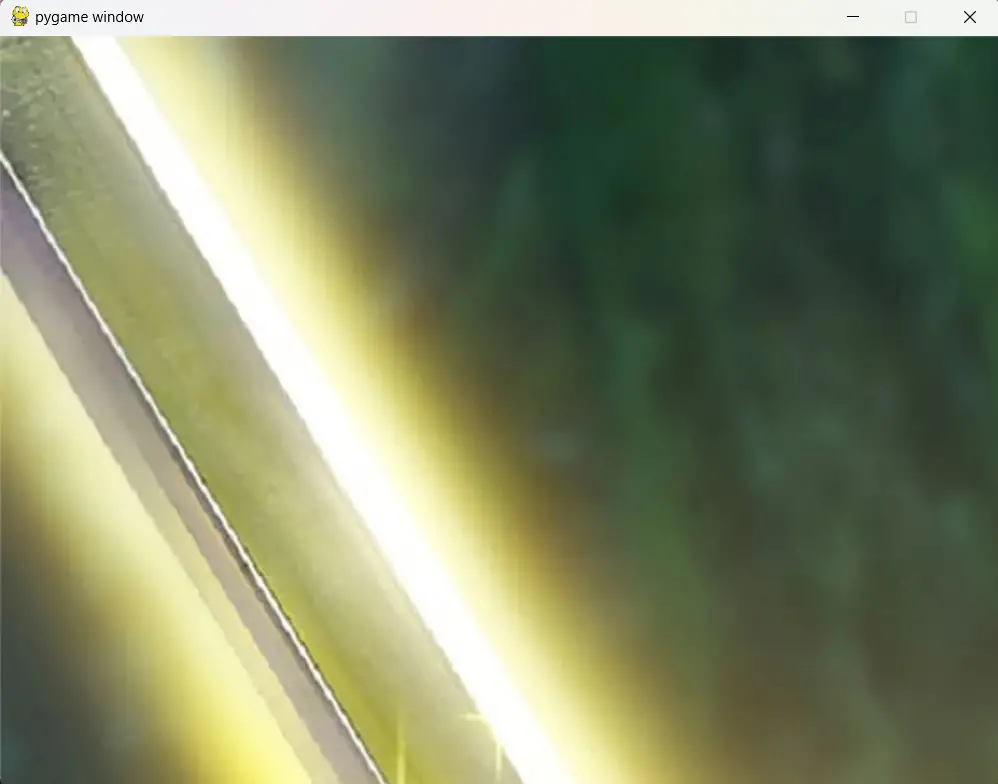
As you can see, it it an incomplete image. This is because the original image was larger than the dimensions of the pygame window. To resolve this, we will auto scale our image down to the size of the pygame window, right after loading it from its url.
import pygame
import requests
import sys
from io import BytesIO
pygame.init()
screen = pygame.display.set_mode((800, 600))
response = requests.get('https://www.denofgeek.com/wp-content/uploads/2022/05/Leged-of-Zelda-Link.jpg')
img = pygame.image.load(BytesIO(response.content))
img = pygame.transform.scale(img, (800, 600)) # <------
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.blit(img, (0, 0))
pygame.display.update()
We now have the correct image.
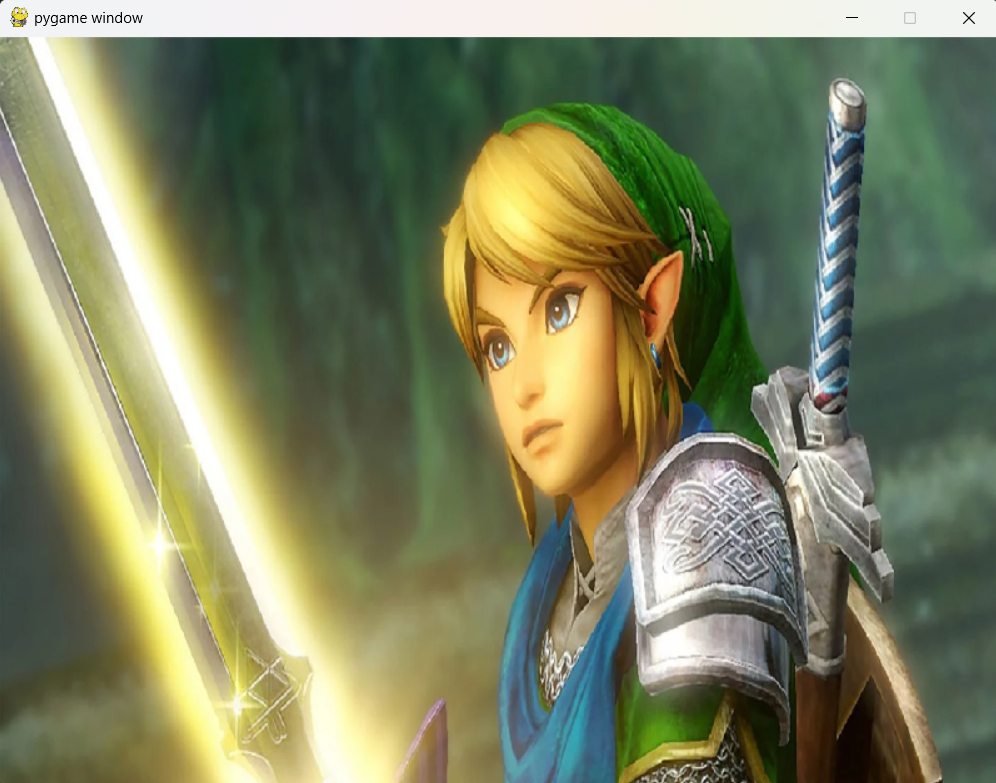
Make sure you don’t put the transformation code in the game loop. That will cause the image to be transformed every iteration, whereas we only need to do it once in the beginning.
You may also want to look into alternatives on how to load images. Other libraries like Pillow support a greater range of image formats, so using them can be beneficial. They also allow for image manipulation operations (more than what pygame has), so that’s another cool benefit.
This marks the end of the “How to Load image from Web URL in Pygame” Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.