Often during the execution of our Matplotlib Program, we will need to update our plot from time to time. Common examples of this are when we need to do plotting in Real-time. In such cases the Plot must be updated very frequently. But how do we do so?
There are two methods that you can use. Let’s discuss them one by one.
Method#1 – Clear and Redraw the Plot
The first method is completely “clear” the plot of all its axis and data, and then redraw it. It’s basically like wiping the Window clean and redrawing everything.
To “clear” the plot, we will first use the clear() method on the axis
object. Now we can make whatever changes we need to, (such as add a few extra values or modify a few existing values in our dataset) then redraw the plot using the plot()
method.
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
fig, ax = plt.subplots()
x = [3, 5, 8]
y = [9, 8, 4]
ln, = ax.plot(x, y, '-')
def update(frame):
global x, y
ax.clear()
x.append(9)
y.append(6)
ln, = ax.plot(x, y, '-')
animation = FuncAnimation(fig, update, interval=2000, repeat = False)
plt.show()
We have used the Animation module in Matplotlib to help us update the data after two seconds. It is a safe and efficient way of scheduling events for Matplotlib graphs (matplotlib is not very compatible with regular threads).
The output of the above code:
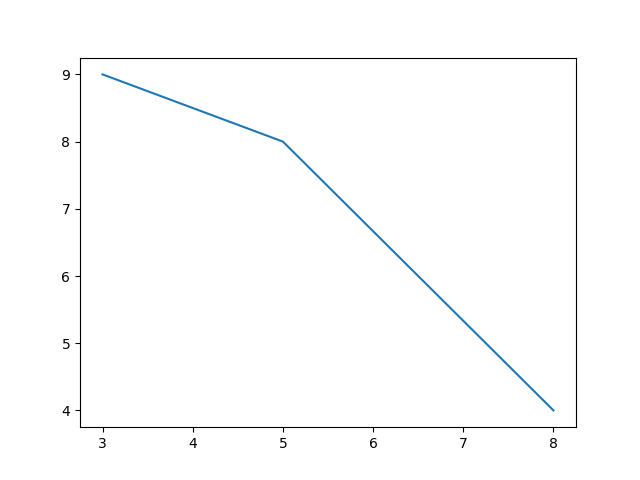
The output after two seconds:
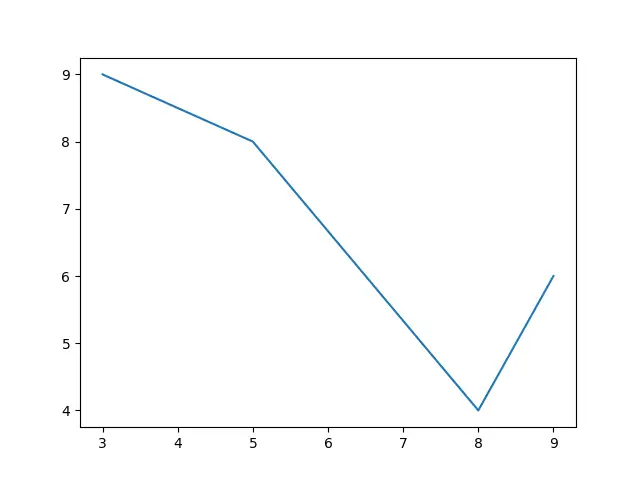
You can clearly see here how we have updated the data in matplotlib during its execution.
Method#2 – Update Data for Plot
There is a better and faster way of updating a plot in matplotlib. This technique does not involve clearing our plot, instead it directly updates the part that need to be updated. It is faster because there is less time wasted in clearing and redrawing things that do not need to be redrawn.
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
fig, ax = plt.subplots()
x = [3, 5, 8]
y = [9, 8, 4]
ln, = ax.plot(x, y, '-')
def update(frame):
global x, y
x.append(9)
y.append(6)
ln.set_data(x, y)
fig.gca().relim()
fig.gca().autoscale_view()
return ln,
animation = FuncAnimation(fig, update, interval=2000, repeat = False)
plt.show()
There are three new lines of code here. The set_data
function updates the “line object” with the new data. This line alone will update the graph, but there are some potential problems that could occur. Since we are not redrawing the whole plot, if the new data exceeds the default axis range, then the plot will go outside the window.
For this we need to call the relim()
and autoscale_view()
functions, which reset the axis ranges and adjusts the size of the window if necessary.
To test this out, remove the two lines with relim()
and autoscale_view()
to see what happens. This is because the new data has a X-value of 9, which exists outside the default range (which was previously from 3 to 8 on the X-axis).
This marks the end of the How to Update a plot in Matplotlib Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.