This article covers the QComboBox widget in PyQt (PyQt5).
A QComboBox widget presents a drop-down list of items for the user to select an option from. The advantage of this widget is that it takes up very little space on the screen, even if it has alot of different options. This is the widget to be used in space restricted PyQt GUI programs.
You can find a complete list of QComboBox methods and options at the end of this tutorial.
Creating a QComboBox
Below is the simplest example of a QComboBox widget in a PyQt GUI.
You can create a Combobox using the QComboBox()
function and passing the window object as a parameter. Next you simply have to add Items to it, from which the user will select one.
Remember, you can only add String values to a Combobox!
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
combo = QtWidgets.QComboBox(win)
combo.addItem("Python")
combo.addItem("Java")
combo.addItem("C++")
combo.move(100,100)
win.show()
sys.exit(app.exec_())
The output for the above code:
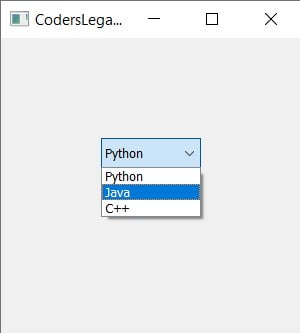
You can use the addItems()
instead to pass a list of different options at once. It’s better to use this generally in order to conserve space.
combo.addItems(["Python", "Java", "C++"])
Retrieving QComboBox Values
We’ve created a QComboBox, but how to find out which option the user picked? Using the currentText()
method on the widget will return it’s current selected value, but how to call this method?
The easiest and most common solution is to use a button that acts as a “Submit” button. The button, when clicked calls a functions that prints the value of the combobox to screen using the currentText()
method.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
def show():
result = combo.currentText()
print(result)
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
combo = QtWidgets.QComboBox(win)
combo.addItems(["Python", "Java", "C++"])
combo.move(100,100)
button = QtWidgets.QPushButton(win)
button.setText("Submit")
button.clicked.connect(show)
button.move(100,200)
win.show()
sys.exit(app.exec_())
Below is a picture of the GUI output of the above code, side by side the Python IDE. We selected each value randomly and clicked the submit button, causing it to be displayed on the console output.
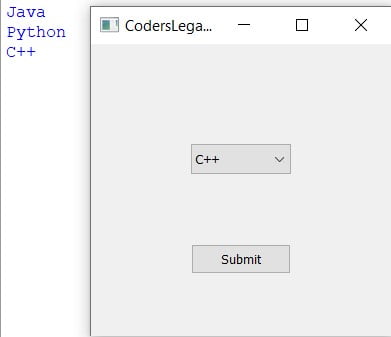
If you wish to display the output on the GUI itself, you can use the QLabel widget to do so.
QComboBox Methods
We covered the most important and common methods in detail above, but here is a complete list of all them.
Method | Description |
---|---|
addItem() | Adds an item. |
addItems() | Adds several items in the form of a list. |
Clear() | Deletes all the items. |
count() | Returns an integer representing the number of items. |
currentText() | Retrieves the text of currently selected item |
itemText(index) | Returns the text at the specified index. |
currentIndex() | Returns the index of the selected option. |
setItemText(index,text) | Changes the text of the item at the specified index. |
setFixedWidth() | Defines the size of the widget in pixels. |
This marks the end of the PyQt QComboBox article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content should be asked in the comments section below.
Head back to the main PyQt5 homepage to learn about the other great widgets.
The use of button may be superfluous, see the following example with ‘currentTextChanged’:
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QComboBox, QLabel
class Finestra(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle(“Esempio QComboBox”)
layout = QVBoxLayout()
self.combo = QComboBox()
self.combo.addItems([“Opzione 1”, “Opzione 2”, “Opzione 3”])
self.label = QLabel(“Seleziona un’opzione”)
self.combo.currentTextChanged.connect(self.aggiorna_label)
layout.addWidget(self.combo)
layout.addWidget(self.label)
self.setLayout(layout)
def aggiorna_label(self, testo):
self.label.setText(f”Hai selezionato: {testo}”)
if __name__ == “__main__”:
app = QApplication([])
finestra = Finestra()
finestra.show()
app.exec_()