This pyqt tutorial explains the use of the pyqt5 QTableView widget.
Tables and Spreadsheets are a very common type of widget/component in GUI windows. Microsoft excel is one such software with spreadsheets that can store values.
PyQt5 provides us with the QTableView widget which can be used to create such spreadsheets and tables. These Tables are created from a combination of rows and columns. The intersection between rows and columns creates cells. Each cell in the TableView widget is editable and can be interacted with (e.g: entering or editing data).
Creating a TableView in PyQt5
In this section we’ll explain how to create a simple TableView widget in PyQt5.
We’ll be going through the process step by step, explaining every line of code.
from PyQt5.QtWidgets import QApplication, QWidget,
QTableWidget, QTableWidgetItem,
QVBoxLayout
import sys
First up we have to make a few imports. We are importing the bare minimum of widgets required to create a simple PyQt5 GUI window and a QTableView widget.
class Window(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQt5 QTableView")
self.setGeometry(500, 400, 500, 300)
self.Tables()
self.show()
This is the Window Class that’s going to be holding all our code. The above code includes the first method, __init__
. It contains some basic initialization code, which sets the title and defines the geometry of our GUI window.
It is also responsible for calling the Tables()
method (in the next example) and displaying the PyQt5 window with the show
method.
def Tables(self):
self.tableWidget = QTableWidget()
self.tableWidget.setRowCount(5)
self.tableWidget.setColumnCount(3)
self.vBox = QVBoxLayout()
self.vBox.addWidget(self.tableWidget)
self.setLayout(self.vBox)
The above code shows the main method in the Window class called Tables
. It first creates the TableView using the QTableWidget
function. Next we set the number of rows and columns using the setRowCount
and setColumnCount
methods on the tableview
object.
The last three lines are for the layout of the GUI window. We’re going to be using the VBox Layout here, although it doesn’t matter much since we only one widget (so far).
App = QApplication(sys.argv)
window = Window()
sys.exit(App.exec())
These are the three lines of code which are located outside of the class. They are responsible for creating the QApplication, the class object and the exit command for the window.
After putting together all the code and executing it, we can see our PyQt QTableView widget as shown below.
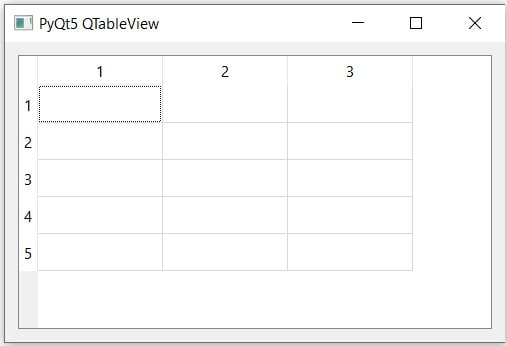
Are you interested in migrating to PyQt6? You can learn how to create the same QTableView in PyQt6 as well!
PyQt QTableView Example
In the previous example we simply created an empty Table with no data. In this example we’ll be creating a PyQt QTableView filled with values as well. You also begin to understand how the layout of the TableView widget works in this example.
This example will mainly be featuring the below method, setItem()
. It takes three parameters, the row index, the column index and a QTableWidgetItem
object.
The row and column index determine the position of the Item in the layout of the Table. See the image below for reference. The QTableWidgetItem
determines the value that is to be stored in that cell.
self.tableWidget.setItem(0,0, QTableWidgetItem("Name"))
You can use the below image as reference for the layout and indexes. It should help build your understanding.
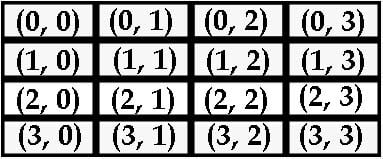
Below is an example with the full required code.
from PyQt5.QtWidgets import QApplication, QWidget,
QTableWidget, QTableWidgetItem,
QVBoxLayout
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQt5 QTableView")
self.setGeometry(500, 400, 500, 300)
self.Tables()
self.show()
def Tables(self):
self.tableWidget = QTableWidget()
self.tableWidget.setRowCount(5)
self.tableWidget.setColumnCount(3)
self.tableWidget.setItem(0,0, QTableWidgetItem("Name"))
self.tableWidget.setItem(0,1, QTableWidgetItem("Age"))
self.tableWidget.setItem(0, 2 , QTableWidgetItem("Gender"))
self.tableWidget.setColumnWidth(0, 200)
self.tableWidget.setItem(1,0, QTableWidgetItem("John"))
self.tableWidget.setItem(1,1, QTableWidgetItem("24"))
self.tableWidget.setItem(1,2, QTableWidgetItem("Male"))
self.tableWidget.setItem(2, 0, QTableWidgetItem("Lucy"))
self.tableWidget.setItem(2, 1, QTableWidgetItem("19"))
self.tableWidget.setItem(2, 2, QTableWidgetItem("Female"))
self.tableWidget.setItem(3, 0, QTableWidgetItem("Subaru"))
self.tableWidget.setItem(3, 1, QTableWidgetItem("18"))
self.tableWidget.setItem(3, 2, QTableWidgetItem("Male"))
self.tableWidget.setItem(4, 0, QTableWidgetItem("William"))
self.tableWidget.setItem(4, 1, QTableWidgetItem("60"))
self.tableWidget.setItem(4, 2, QTableWidgetItem("Male"))
self.vBox = QVBoxLayout()
self.vBox.addWidget(self.tableWidget)
self.setLayout(self.vBox)
App = QApplication(sys.argv)
window = Window()
sys.exit(App.exec())
The GUI output of our new Python QTableView widget:
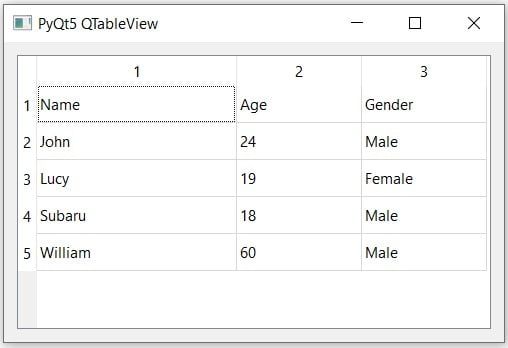
You can further extend the functionality by adding a few button widgets as well, which will act as “save” and “load” buttons. This will allow you to save any changes you make to the TableView as well as load the data into the table later.
This marks the end of the PyQt QTableView with PyQt5 Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section.