This article covers the file dialog in PyQt5.
File Dialogs are an important part of any software that involves a GUI. Whether it’s your operating system or a little GUI program you’ve developed, File Dialogs have a great number of uses, most important which is the ability to have the user select/save a File on the File Path of his choice.
PyQt5 introduces the QFileDialog widget allows us to create a variety of different File dialogs such as QFileDialog.getOpenFileName(), QFileDialog.getOpenFileNames() and QFileDialog.getSaveFileName().
getOpenFileName Dialog
Pass None
into the first parameter, and in the second pass the Title you want to give your File Dialog. In the final parameter you pass the file types that you want to see. You can put multiple options by separating them with ";;"
. We have inserted 3 possible selections in the below code.
Another thing to note is that the File Dialog function returns two parameters. The first is the File path of the selected file and the second is a Boolean value depending on whether a file was selected or not.
(in some cases you may have to pass self in the first parameter if you’re using a class based approach)
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QFileDialog
from PyQt5 import QtCore
import sys
def dialog():
file , check = QFileDialog.getOpenFileName(None, "QFileDialog.getOpenFileName()",
"", "All Files (*);;Python Files (*.py);;Text Files (*.txt)")
if check:
print(file)
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
button = QPushButton(win)
button.setText("Press")
button.clicked.connect(dialog)
button.move(50,50)
win.show()
sys.exit(app.exec_())
The output of the above code:
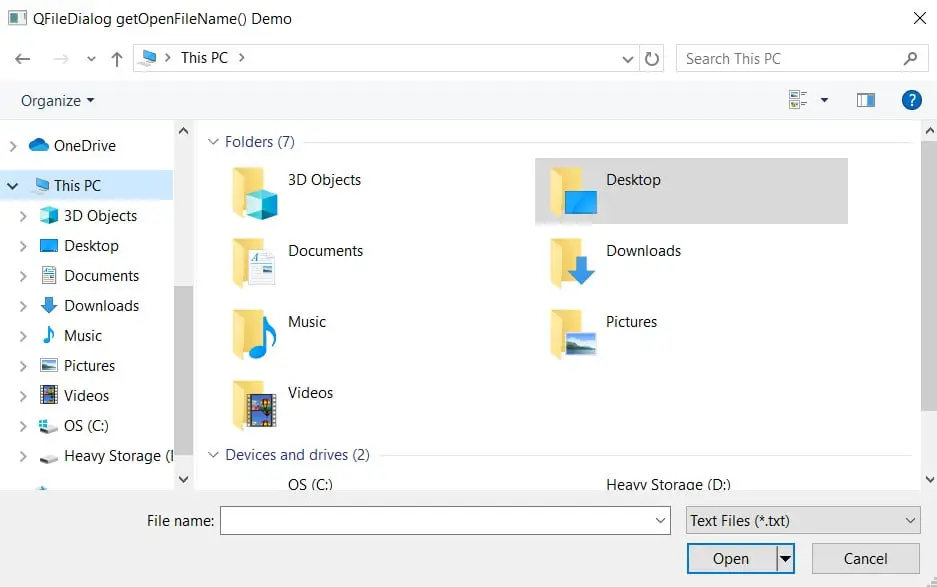
Once the above window appeared, navigating to the Desktop and selecting a Python file from there returned the following string.
C:/Users/CodersLegacy/Desktop/Game.py
You can toggle what kind of file types will appear to be selected through the little drop-down combobox in the bottom right corner. If you want to change the possible options here, modify the final parameter of the File dialog function.
Here’s a closer look at the possible file types that will show up. You can switch between the three of these.
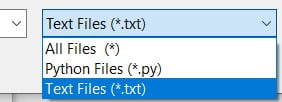
getOpenFileNames Dialog
There is another FileDialog very similar to this one called QFileDialog.getOpenFileNames()
, the only difference being an additional “s”. This File Dialog allows you to select multiple Files at once by holding down the CTRL key and clicking on them.
If you select multiple files, their file paths are returned of a list containing strings. The look of the dialog is the exact same so there isn’t any need for an image.
getSaveFileName
All File dialogs have 90% similarities. They are all designed to return strings regarding File paths in one way or the other. The only difference is in the look of the Dialog.
def dialog():
file , check = QFileDialog.getSaveFileName(None, "QFileDialog getSaveFileName() Demo",
"", "All Files (*);;Python Files (*.py);;Text Files (*.txt)")
if check:
print(file)
The position of the Combobox (drop down selection) has changed and the text on one of the buttons has changed from Open to Save.
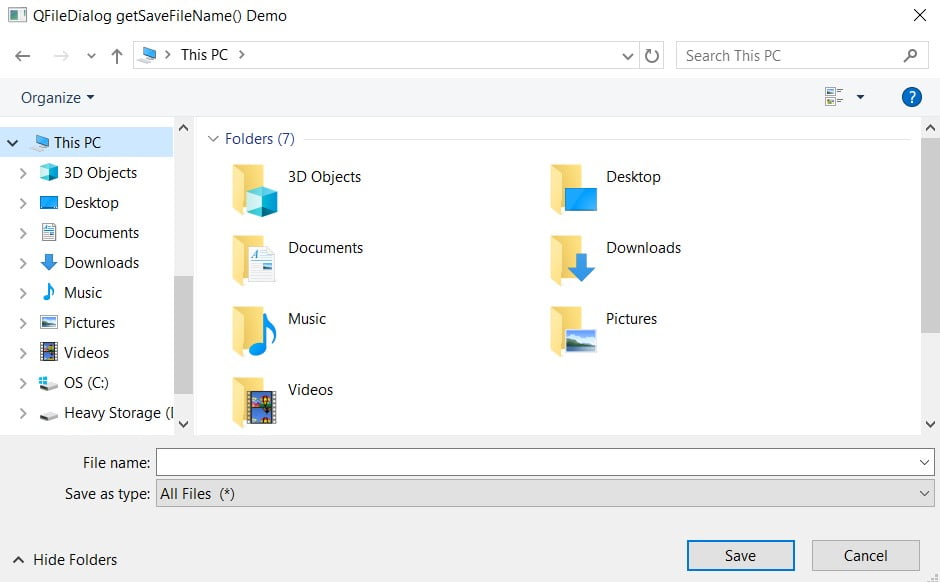
There are more File Dialogs than these available out there. We simply covered the most commonly used ones. If you want to learn about the others, you can follow this link to the documentation.
This marks the end of the PyQt5 File Dialog article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.
Check out other Dialogs in PyQt5:->
QInputDialog