This article covers the PyQt6, QLabel widget.
QLabel is a basic “bread and butter” kind of widget, used to display lines of text on the PyQt6 GUI window. This widget comes bundled with many supporting functions and methods, allowing us to retrieve and update the displayed text easily.
A list of helpful methods for the QLabel can be found at the bottom of the tutorial.
Creating a QLabel in PyQt6
Let’s start with a simple example which will demonstrate how to create a simple QLabel Widget in PyQt6.
from PyQt6.QtWidgets import QApplication, QWidget, QLabel
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.resize(300, 250)
self.setWindowTitle("CodersLegacy")
label = QLabel("GUI Applications with PyQt6", self)
label.move(80, 100)
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
The output of the above code is shown below.
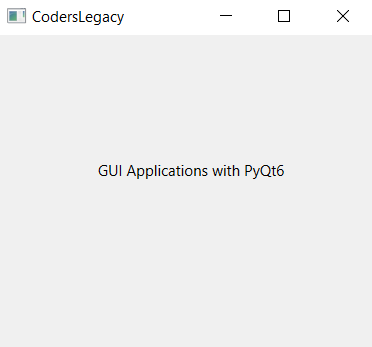
Let’s proceed to examine the above code line by line, with a proper explanation.
from PyQt6.QtWidgets import QApplication, QWidget, QLabel
import sys
Basic imports that we need for our application. All widget Classes like QLabel can be found in the QtWidgets module within PyQt6.
class Window(QWidget):
def __init__(self):
super().__init__()
Here we are creating a Class where we will be writing all our code. To give it the functionality of a QWidget, we make it inherit from QWidget. We also need to execute super().init()
, in order to execute the constructor of the Parent Class (QWidget).
self.resize(300, 250)
self.setWindowTitle("CodersLegacy")
These are two functions that our window can now use thanks it’s inheritance from QWidget. The first resizes the window to dimensions 300×250. The second sets the Title that appears on the top-left corner.
label = QLabel("GUI Applications with PyQt6", self)
This creates the QLabel widget. The first parameter is the Text we want to display on the QLabel. The second parameter is the parent widget in which we want to place the widget.
label.move(80, 100)
Here we are moving the Label widget from it’s default position to (0, 0) on the screen to the 80th X-coordinate and the 100th Y-coordinate.
Alternatively, you can center the label on the Screen in a more proper fashion using setAlignment()
. This function takes an enumerable called AlignmentFlag
from the Qt Module, which contains flags like AlignCenter, AlignRight, AlignLeft, etc. (An example of this is shown in the next section)
move()
is a very basic layout system, and not suitable for actual projects. PyQt6 comes with actual layouts, in charge of managing positions of the widgets inside the window. Learn more about them in our PyQt6 layout management tutorial.
Retrieving and Updating QLabel values
In this section we’ll explore how to change the currently displayed text on the QLabel Widget, and how to retrieve the currently displayed text on the QLabel. To do so, we require the use of the QPushButton widget which will call the functions which contain the required methods that we will use on QLabel.
To update the value of a Label, we use the setText()
method, and we use text()
to get the current text value. We will create two new functions in our Class, which we will link our buttons to. These functions will be called whenever the buttons are pressed.
We will be using a Layout Manager in the below code to organize our widgets.
from PyQt6.QtWidgets import (
QApplication, QVBoxLayout, QWidget, QLabel, QPushButton
)
from PyQt6.QtCore import Qt
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.resize(300, 250)
self.setWindowTitle("CodersLegacy")
layout = QVBoxLayout()
self.setLayout(layout)
self.label = QLabel("Old Text")
self.label.setAlignment(Qt.AlignmentFlag.AlignCenter)
self.label.adjustSize()
layout.addWidget(self.label)
button = QPushButton("Update Text")
button.clicked.connect(self.update)
layout.addWidget(button)
button = QPushButton("Print Text")
button.clicked.connect(self.get)
layout.addWidget(button)
def update(self):
self.label.setText("New and Updated Text")
def get(self):
print(self.label.text())
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
Remember to declare the labels using self.
, otherwise you won’t be able to use them in other functions of the same class.
Furthermore, we don’t need to specify the parent for the widget anymore since we have already placed it within the layout using addWidget
, which has been placed inside the Window using setLayout
.
The Output:
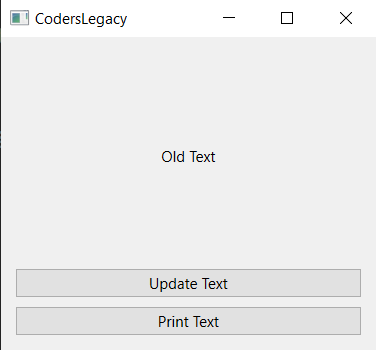
After Clicking the Button:
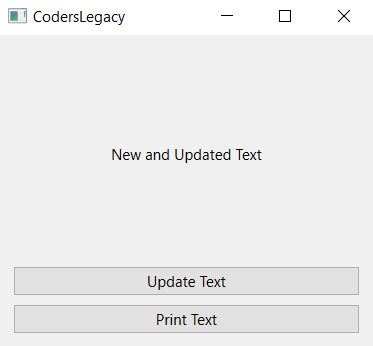
If you are interested in learning how to customize the label text, check out our tutorial on “PyQt6 Fonts”.
QLabel Methods
A compilation of the most important methods available for the Label widget.
Method | Description |
---|---|
setAlignment() | Decides the Alignment of the text. Some of the possible parameters: Qt.AlignmentFlag.AlignLeft, Qt.AlignmentFlag.AlignRight, Qt.AlignmentFlag.AlignCenter, Qt.AlignmentFlag.AlignJustify, Qt.AlignmentFlag.AlignBottom and Qt.AlignmentFlag.AlignTop. |
setIndent() | Defines the indentation for the text. |
setPixmap() | Used to display an image. |
text() | Returns the (displayed) text value for the label. |
setText() | Sets the text that is displayed on the widget. |
selectedText() | Returns the text that has been selected by the user. (For this to work, textInteractionFlag must be set to TextSelectableByMouse). |
setWordWrap() | Used to enable or disable text wrapping. |
adjustSize() | Automatically adjusts the Label size to the text being displayed. |
This marks the end of the PyQt6 QLabel widget article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.