This article covers the PyQt6 QPushButton widget with examples.
One of the most basic and common widgets in PyQt6. Using the QPushButton Class, we can create a button, that we then link to a function. Clicking on the button will cause the linked function to execute. Of course, that function will contain whatever code we wish to execute whenever the button is triggered. (Such as printing some text to the console)
You will find a list of all the methods for the PyQt6 QPushButton available at the bottom of this page.
Creating a QPushButton Widget
From the QtWidgets Module in PyQt6, we must import the QPushButton
Class, which we must use to create Button objects. We can then interact this button object with methods like setText()
and move()
, or customize it using CSS Stylesheets.
from PyQt6.QtWidgets import QApplication, QWidget, QPushButton
from PyQt6.QtCore import Qt
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.resize(250, 250)
self.setWindowTitle("CodersLegacy")
button = QPushButton("Hello World", self)
button.move(100, 100)
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
Below is the output of the code we just wrote. We haven’t mapped this button to any function yet, so clicking it won’t do anything.
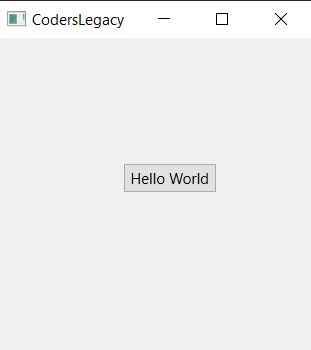
move()
is a very simplistic way of managing widget positions, and not suitable for actual projects or when dealing with a large number of widgets. PyQt6 comes with actual layout managers, in charge of managing positions of the widgets inside the window. Learn more about them in our PyQt6 layout management tutorial.
Connecting Buttons to Functions
A button which isn’t connected to a function is a useless button. Buttons are meant to be connected to a function which will execute once the button has been pressed.
We’ll explore several popular usages of the QPushButton widget in PyQt5, such as closing the window, updating text and retrieving widget values.
Updating Text on a Label
In this example we will be using a Button to change the text on a Label. You can learn more about the PyQt6 Label and it’s methods in it’s own dedicated tutorial.
The main takeaway here is to see how we can practically use a Button to perform a task. We will make use of the “Signal System” in PyQt6. Signals are basically indicators that indicate that certain events have occurred, such as a button click, or some text being modified. We will be using the clicked
signal that the Button emits whenever it is clicked.
The format for signals is as follows:
widget.signal_name.connect(function_name)
Function name refers to the function that we want to connect to the signal. So whenever that signal is connected, the function connected to it will run.
from PyQt6.QtWidgets import (
QApplication, QVBoxLayout, QWidget, QLabel, QPushButton
)
from PyQt6.QtCore import Qt
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.resize(300, 250)
self.setWindowTitle("CodersLegacy")
layout = QVBoxLayout()
self.setLayout(layout)
self.label = QLabel("Old Text")
self.label.setAlignment(Qt.AlignmentFlag.AlignCenter)
self.label.adjustSize()
layout.addWidget(self.label)
button = QPushButton("Update Text")
button.clicked.connect(self.update)
layout.addWidget(button)
button = QPushButton("Print Text")
button.clicked.connect(self.get)
layout.addWidget(button)
def update(self):
self.label.setText("New and Updated Text")
def get(self):
print(self.label.text())
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
The Output:
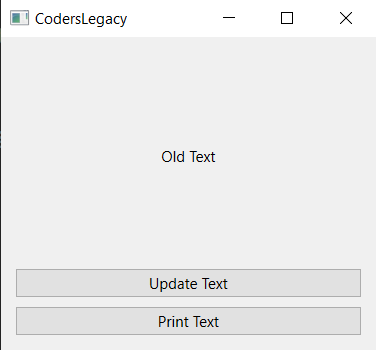
After Clicking the Button:
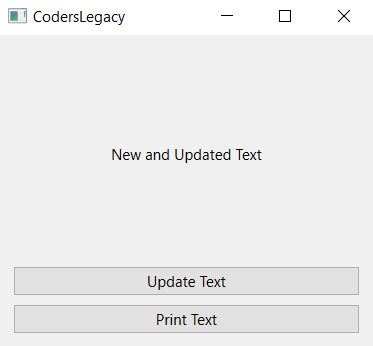
How to Close a PyQt6 application
Another popular usage of QPushButton is to create a “Quit Button” that closes the PyQt6 application when clicked. Here’s how to do it.
We simply use the close()
method on the window that we created using the QMainWindow()
function.
Instead of creating a whole separate function and then calling win.close()
, we can take a shortcut and simply pass win.close
to the connect function. Remember to leave off the parenthesis, else the function will call itself automatically.
We’ve also thrown in an extra method, called resize()
which is used to change the size of the QPushButton. The first parameter defines the new width, and the second defines the new height.
from PyQt6.QtWidgets import QApplication, QWidget QPushButton
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.resize(300, 250)
self.setWindowTitle("CodersLegacy")
button = QPushButton("Quit Button", self)
button.clicked.connect(self.close)
button.resize(120,60)
button.move(160, 180)
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
The Output:
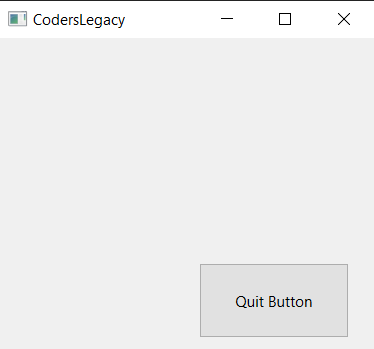
Adding an Image to QPushButton
You can set an image onto your button to make it look more stylish using the QIcon widget. Just pass the filepath into it and you’re done. Remember to make the correct import, and use the setIcon()
method on the Button object.
from PyQt6.QtGui import QIcon
button.setIcon(QIcon('myImage.jpg'))
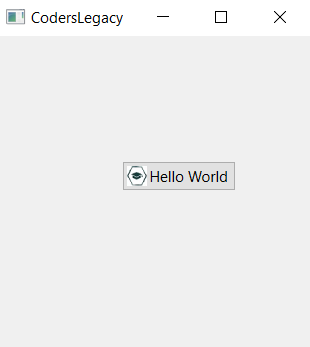
QPushButton Methods
This is a compilation of all important and common methods for the QPushButton widget.
Method | Description |
---|---|
isDefault() | A button with this property set to true (i.e., the dialog’s default button,) will automatically be pressed when the user presses enter |
setIcon() | Displays an image icon on the button. |
setEnabled() | True by Default. If set to False, but will become grey and unresponsive. |
setText() | Sets the displayed text on the button. |
text() | Returns the displayed text on the button. |
toggle() | Toggles the state of the button. |
Want to learn more about PyQt6? Head over to our PyQt6 Section for more dedicated tutorials!
This marks the end of the PyQt6 QPushButton widget article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.