What is the Python Tkinter Combobox?
A special extension of Python Tkinter, the ttk
module brings forward this new widget. The Python Tkinter Combobox presents a drop down list of options and displays them one at a time. It’s good for places where visibility is important and has a modern look to it.
The Python Combobox is actually a sub class of the widget Entry
. Hence it inherits many options and methods from the Entry
class as well as bringing some new ones of it’s to the table.
ComboBox Syntax
You must specially import the ttk module to be able to use Comboboxes.
from tkinter import ttk
Combo = ttk.Combobox(master, values.......)
Some important Combobox options are listed below.
No | Option | Description |
---|---|---|
1 | exportselection | Boolean value. If set, the widget selection is linked to the Window Manager selection |
2 | justify | Specifies how the text is aligned within the widget. One of “left”, “center”, or “right”. |
3 | height | Specifies the height of the pop-down listbox, in rows. |
4 | postcommand | A script that is called immediately before displaying the values. It may specify which values to display. |
5 | state | One of “NORMAL, “READONLY”, or “DISABLED”. Normal is the default state. Readonly prevents the user from editing any values. disabled shuts down the widgets and greys it out. |
6 | textvariable | Specifies a name whose value is linked to the widget value. Whenever the value associated with that name changes, the widget value is updated, and vice versa. |
7 | values | Specifies the list of values to display in the drop-down listbox. |
8 | width | Specifies an integer value indicating the desired width of the entry window, in average-size characters of the widget’s font. |
Assigning a ComboBox Values
In the example we create a list of values, and then feed them to the values
option in the Combobox.
By Default the Combobox is spawned with no value selected. In other words, it’s blank. If you wish, you can avoid this using the set()
function.
from tkinter import *
from tkinter import ttk
root = Tk()
root.geometry("200x150")
frame = Frame(root)
frame.pack()
vlist = ["Option1", "Option2", "Option3",
"Option4", "Option5"]
Combo = ttk.Combobox(frame, values = vlist)
Combo.set("Pick an Option")
Combo.pack(padx = 5, pady = 5)
root.mainloop()
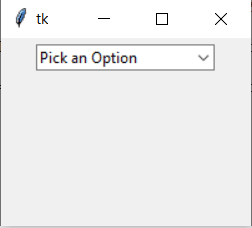
If the ComboBox was not what you were looking for, it has a variant called the SpinBox which may interest you.
Retrieving Combobox values
Once the user has selected an option, we need a way to retrieve his input. For this we need a button which the User must trigger. The button calls a function that uses the get()
function to retrieve the current value of the Combobox.
from tkinter import *
from tkinter import ttk
def retrieve():
print(Combo.get())
root = Tk()
root.geometry("200x150")
frame = Frame(root)
frame.pack()
vlist = ["Option1", "Option2", "Option3",
"Option4", "Option5"]
Combo = ttk.Combobox(frame, values = vlist)
Combo.set("Pick an Option")
Combo.pack(padx = 5, pady = 5)
Button = Button(frame, text = "Submit", command = retrieve)
Button.pack(padx = 5, pady = 5)
root.mainloop()
Video Code
The Code from our Video on Tkinter ComboBox Widget on our YouTube Channel for CodersLegacy.
import tkinter as tk
from tkinter import ttk
class Window:
def __init__(self, master):
self.master = master
# Frame
self.frame = tk.Frame(self.master, width = 200, height = 200)
self.frame.pack()
self.vlist = ["Option1", "Option2", "Option3", "Option4", "Option5"]
self.combo = ttk.Combobox(self.frame, values = self.vlist, state = "readonly")
self.combo.set("Pick an Option")
self.combo.place(x = 20, y = 50)
root = tk.Tk()
root.title("Tkinter")
window = Window(root)
root.mainloop()
This marks the end of the Tkinter Combobox article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.
You can head back to the main Tkinter article using this link.