Strings are one of the most basic data types in Python, however they have dozens of functions associated with them, increasing functionality and flexibility. In order to have full control over strings in Python, you need to learn them all.
What is a String?
A string is a simple piece of text enclosed within double quotation marks. Below is an example of a string being assigned to a variable.
var = "Hello World"
String Concatenation
To “concat” means to join together. In Python, two strings can be concatenated together with the “+” operator as shown below.
var = "Hello"
var2 = "World"
var3 = var + var2
print(var3)
"Hello World"
If you want to add a number to a string, first convert it to a string.
var = "Hello world"
num = 4
print(var + str(num))
"Hello world 4"
Iterating through a string
If you haven’t studied for loops yet, you can ignore this section. This section will also be present on the for loops page for you to check out later.
a = "Hello World"
for x in a:
print(x)
What this does is iterate through every individual character in the string and print it out. You can expect an output like this.
H
e
l
l
o
W
o
r
l
d
String Indexing
Python treats each character in a string as an individual data type. It has no data type for characters, hence a character is just a string with length 1. Python strings are thus a collection of many of these small strings, or in other words they are a “list” or “array” of characters.
Being treated as individual data types gives us greater flexibility, allowing us to access each character individually. (Remember, in Python, Indexes start from zero)
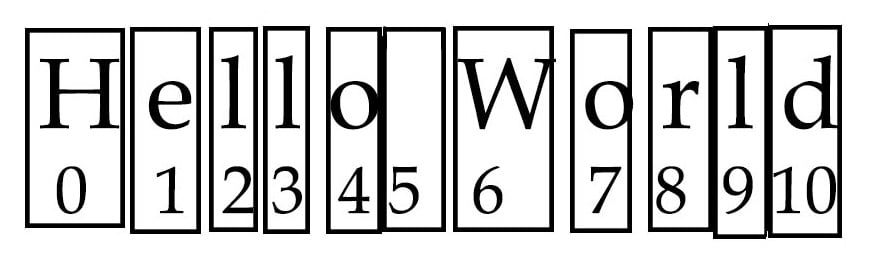
var = "Hello World"
print(var[1])
# Output
e
We can also splice parts of strings. For instance, if we want the first 5 characters in a string.
var = "Hello World"
print(var[0:5])
# Output
Hello
Another feature in string indexing is negative indexing. If the number with the square brackets is negative, then the string will start counting the characters from the end of the string rather than the start.
var = "Hello World"
print(var[0]) # Outputs "H"
print(var[-1]) # Outputs "d"
String Membership
If you want to find out whether a specific word is in the large piece of text, or something similar, there’s a quick and easy way of doing so.
var = "Hello World. This is Coder's Legacy"
if "World" in var:
print("Found")
Common String Functions
lower()
lower()
converts all uppercase characters into lowercase. Insert a string or suitable variable into it’s parameters to convert.
var = "HELLO WORLD"
print(lower(var)) # Outputs "hello world"
upper()
The reverse of the lower()
Function. Converts all lowercase characters to uppercase characters.
var = "hello world"
print(lower(var)) # Outputs "HELLO WORLD"
swapcase()
Swaps a lower case character for an uppercase character and vice versa.
var = "HELLO world"
print(swapcase(var)) # Output: "hello WORLD"
split()
The split()
function, “splits” the string around the character given in the parameters. The character in the parameters of the split() function is known as the separator. Default separator is white space.
var = "Hello World"
print(var.split()) # Outputs ["Hello" , "World"]
var = "A#B#C"
print(var.split('#')) # Outputs ["A","B","C"]
Keep in mind the output of the split()
function is of type list, not string.
strip()
The strip()
function removes all white spaces at the start or ending of the string.
var = " Hello World "
print(var.strip()) # Outputs "Hello World"
len()
Calculates and outputs the length of a string. A string with 5 characters, has length 5. White spaces in strings will count as characters as well.
var = "Hello World"
print(len(var))
replace()
This function replaces a character or string with another character or string. replace()
takes two inputs as parameters. The string to be replaced and the new string.
Remember that uppercase and lowercase characters have different values.
var = "Hello World"
print(replace("Hello","Goodbye")) #Outputs, "Goodbye World")
startswith()
The startswith()
function checks whether a string is starting with a specific prefix (string).
var = "This is a Coder's Legacy"
result = var.startswith("This is")
result2 = var.startswith("Coder's")
print(result)
print(result2)
Output will be:
True
False
count()
A simple function that takes a string as parameter, and outputs the number of times it appeared within the target string.
var = "Hello World. Hello World. Hello World"
result = var.count("Hello")
print(result)
Output will be:
3
Other String Functions:
capitilize()
: Converts only the first character of a string to uppercase.
center():
Takes a number as input, and proceeds to center the target string along that number’s length.
encode()
: Produces an encode version of the target string. Default encoder is UTF-8.
endswith()
: Returns true if the target string ends with a specific string (The string that is taken as a parameter).
This marks the end of the Python Strings Article. Any suggestions or contributions for CodersLegacy are more than welcome. Any questions can be directed to the comments section below.