We’ll be covering the basic rules that outline how python is be written. In the world of programming, these rules are referred to as syntax. In this, the rules defining Python are known as “Python Syntax”.
When these rules are not being obeyed, your code will stop running when it encounters the incorrect line of code, and throws a “syntax error”.
Files names:
All python files must be saved with extension .py to be acknowledged as python code. Often, if you’re using an IDE, it will create a file with the .py by itself.
Sometimes however, you may wish to simply create a text file, and replace it’s .txt extent with the .py, converting it into a python file.
Indentation:
An “indent” refers to a space at the beginning of a line or code. You can use either spaces or the “tab” button to indent your code. Most IDE’s will take care of this for you though (once you move to the next line).
Indentation is an important part of Python’s structure. Unless your code is properly indented, it will not run and instead, throw a syntax error. Most IDE’s will point out any indentation errors and even auto indent when you press enter.
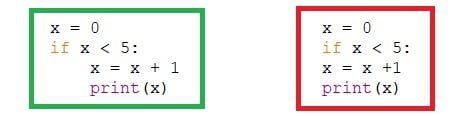
Green shows the correct approach, whereas Red shows the incorrect one.
Note: Indentation is typically used to show a block of code, such as a loop or function.
Comments:
Comments are lines of explanatory text written alongside code. These lines are marked by a special character so that these they aren’t run during execution of the program.
Another integral part of programming, not just Python Syntax, comments. When making large scale applications code can range anywhere from several hundred lines to several thousand, and take many months. Often there are multiple people working on a single project, and they need to be able to write the code in such a way that the others are able to understand it.
Comments are also an integral part of making documentation. So unless you’re building something really small and simple, remember to add comments.
First type of comment, and the most common, add a “#” before the line. Keep in mind though, that this only works on a single line.
# This code will print values of X from 1 to 5
x = 0
while x < 5:
x = x + 1
print(x)
Now maybe you have several lines of text at one point, and adding a “#” sign before them seems too hectic, we have a multi line comment option.
""" This code will
print values of X
from 1 to 5 """
x = 0
while x < 5:
x = x + 1
print(x)
What this basically does if convert the whole paragraph into a string. Python will read this string, but will not execute anything. Hence it functions as a comment.
Variables
In programming, a variable is a value that can change, depending on conditions or on information passed to the program.
Many programming languages require the variable “type” to be declared before a value is assigned to it. This is not the case in Python however. In python you can directly assign a value to a variable, with the variable taking on the type of the first value entered in it.
Note: once the variable type is assigned, you can’t change it by re-assigning a different type value.
If you look at the examples above, we assigned the value 0 to the variable x. What this also did was the give x the type of integer (number), and the value of 0.
Note: This declaration was important as x could not have been used in the loop without a prior value. Any attempt to access a variable that has not been assigned a value will result in a assignment error.
Other important point to keep in mind, is that Variable names cannot begin with a digit. There are some special characters however, (besides alphabets) that can be used in the start, such as underscore.
1var = 0 # INVALID
$var = 0 # INVALID
&123 = 0 # INVALID
var = 0 # VALID
_var = 0 # VALID
There are many naming conventions used for the naming of variables. One such naming convention is Camelcase.
Spelling:
Python is a language whose syntax is case sensitive. If you accidentally end up swapping a lower case letter for a upper case letter or vice versa, you will run into a Syntax error.
Another instance where spelling is important is the naming of variables. The variable “CAT” and “cat” are not the same. Even if it’s just one character different, in any way, it will count as a different variable.
Note: Python is almost always uses lower case
This marks the end of the Python Syntax Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.