Taking input from the user through the console is a very easy task. But what about taking text input from the user through a GUI created in Pygame? This is a bit harder, because Pygame offers no built-in widget that does this for you.
It does however have a bunch of others features that we can use to ease the process and create our method.
Take Text Input from User
First let’s write some basic code to setup our Pygame application.
import pygame
import sys
pygame.init()
display = pygame.display.set_mode((400, 300))
while True:
mouse_pos = pygame.mouse.get_pos()
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
pygame.display.update()
This is the standard code found in pretty much every single pygame application. Let’s begin implementing a technique to get input from the user.
The first thing we will do is setup three new variables. One of these is the font
, which specifies the font family and font size for our text input. Second is text_value
which is a string that we will store in the input in as it gets entered. The third variable text
is a surface representation of the text string, and can be drawn to the pygame screen.
font = pygame.font.SysFont("Verdana", 20)
text_value = ""
text = font.render(text_value, True, (255, 255, 255))
With these values setup, let us turn to the game loop.
Handling Events
In the game loop we need to begin handling the TEXTINPUT
event. This event was introduced in Pygame 2.0, so make sure you have your pygame updated. This event only registers on appropriate keys, and filters out the unwanted ones like “shift” and “ctrl” which we don’t need in our input.
By using the .text
attribute on an event triggered by a key, we can retrieve its text value. We will then concatenate this into the current text value. We will then re-render the text object too.
while True:
mouse_pos = pygame.mouse.get_pos()
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
if event.type == pygame.TEXTINPUT:
text_value += event.text
text = font.render(text_value, True, (255, 255, 255))
display.fill((0, 0, 0))
display.blit(text, (100, 150))
pygame.display.update()
Here we also need to call the fill()
method display. You may not notice it now, but later when we implement “backspace”, you will notice that the characters will not removed without calling fill()
. This is because once something is drawn, it cannot be removed. We need to re-render not only the text, but the entire screen to remove the old changes.
We still aren’t done though. There are two other events that we need to handle as well.
We need to implement the “Backspace” feature. When the user presses the backspace key, the last character should be removed from the input. Secondly, the “Enter/Return” key needs to be handled. Normally when the user presses enter, or clicks a button that text-input is “submitted”. Since we don’t have anywhere to submit it, we will just print it out.
while True:
mouse_pos = pygame.mouse.get_pos()
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_BACKSPACE:
text_value = text_value[:-1]
text = font.render(text_value, True, (255, 255, 255))
if event.key == pygame.K_RETURN:
print(text_value)
if event.type == pygame.TEXTINPUT:
text_value += event.text
text = font.render(text_value, True, (255, 255, 255))
And with this we are done!
The Complete Code
Here is the complete compiled code.
import pygame
import sys
pygame.init()
display = pygame.display.set_mode((400, 300))
background = pygame.Surface((400, 300))
font = pygame.font.SysFont("Verdana", 20)
text_value = ""
text = font.render(text_value, True, (255, 255, 255))
while True:
mouse_pos = pygame.mouse.get_pos()
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_BACKSPACE:
text_value = text_value[:-1]
text = font.render(text_value, True, (255, 255, 255))
if event.key == pygame.K_RETURN:
print(text_value)
if event.type == pygame.TEXTINPUT:
text_value += event.text
text = font.render(text_value, True, (255, 255, 255))
display.blit(background, (0, 0))
display.blit(text, (100, 150))
pygame.display.update()
Here in the output you can see the input that we type being displayed on-screen in the center. (This is because we drew it in the center of course)
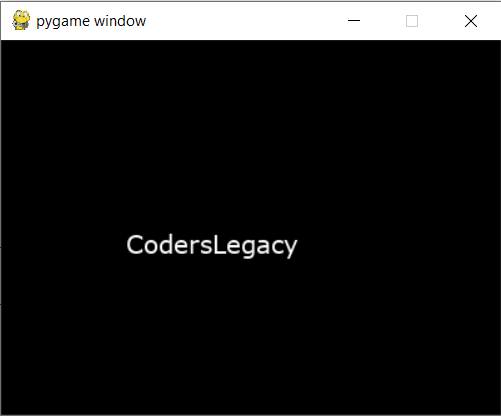
If you want to further expand on this concept and take it a step further, you can take a look at our “Create a Text-Input Box in Pygame” Tutorial. It explores how to create a proper Text-Input-Box Class with the appropriate functions, events and validation checks.
The benefit of such an approach is that you can quickly create multiple widgets without having to manage widget individually.
This marks the end of the Text Input in Pygame Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.
omg so helpfull