This article explains how to close the tkinter window
There are many different ways to close a Tkinter window. The easiest and simplest way is to click the "X"
button on the window. However, this method is rather crude and not very suitable for all situations that you may come across.
Here we’ll be exploring methods which allow you greater control over how your program exits and closes the Tkinter window. We’ll be covering the use of two functions, quit()
and destroy()
used for this purpose.
Destroy Function
The destroy function will cause Tkinter to exit the mainloop()
and simultaneously, destroy all the widgets within the mainloop()
.
from tkinter import *
def close():
root.destroy()
root = Tk()
root.geometry('200x100')
button = Button(root, text = 'Close the window', command = close)
button.pack(pady = 10)
root.mainloop()
The window produced by the above code. Clicking the button will destroy the window, causing it to disappear along with any other widgets in it.
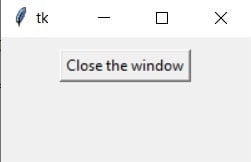
You can also optimize the above code by removing the function, and directly having the button call the destroy function on root
.
from tkinter import *
root = Tk()
root.geometry('200x100')
button = Button(root, text = 'Close the window', command = root.destroy)
button.pack(pady = 10)
root.mainloop()
When you use command = root.destroy
you pass the method to the Button
without the parentheses because you want Button
to store the method for future calling, not to call it immediately when the button is created.
Bonus fact, the destroy function works on more than just the Tkinter window. You can use it on individual widgets as well. Try running the following code and see for yourself.
from tkinter import *
def destroy():
button.destroy()
root = Tk()
root.geometry('200x100')
button = Button(root, text = 'Close the window', command = destroy)
button.pack(pady = 10)
root.mainloop()
You can learn more about the destroy()
function in it’s own dedicated tutorial.
Quit Function
The quit()
function is rather confusing, because of which it’s usually a better (and safer) idea to be using the destroy()
function instead. We’ll explain how to use the quit()
function to close a tkinter window here anyway.
The quit()
function will exit the mainloop, but unlike the destroy()
function it does not destroy any existing widgets including the window. Running the below code will simply stop the TCL interpreter that Tkinter uses, but the window (and widgets) will remain.
from tkinter import *
root = Tk()
root.geometry('200x100')
button = Button(root, text = 'Close the window', command = root.quit)
button.pack(pady = 10)
root.mainloop()
The quit function can cause problems if you call your application from an IDLE. The IDLE itself is a Tkinter application, so if you call the quit function in your app and the TCL interpreter ends up getting terminated, the whole IDLE will also be terminated.
For these reasons, it’s best to use the destroy function.
Related Articles:
This marks the end of the Tkinter close window article. Any suggestions or contributions for Coderslegacy are more than welcome. Questions regarding the article can be asked in the comments section below.