A Modal dialog box is a special type of dialog box or “pop up window” that prevents the user from interacting with the rest of the program until the dialog box is closed. In this tutorial will explore how to create such a modal dialog box in Tkinter.
Tkinter Modal Dialog box (Custom)
The approach that we will take here is to create a Custom Modal Class that we can customize as we wish. Once we have created the Modal Class, we reuse it as many times as we wish without having to write an extra code (other than the single line for creating it).
Shown below is our custom Modal Class. (pretty short, right?)
class Modal(tk.Toplevel):
def __init__(self, master, *args):
tk.Toplevel.__init__(self, master, *args)
self.title("Modal")
tk.Label(self, text = "This is a Modal").pack(padx=30, pady=20)
self.grab_set()
All we had to do was make it inherit from TopLevel (because we want a separate window). We then gave it a custom title, added a label widget to display some text.
The last line is actually pretty important. The “grab_set
()” method is used to explicitly transfer focus to the window it is called on. This focus can only be explicitly removed (by closing the window, or calling grab_set()
on another function. (We cannot interact with any other window until the window with grab_set has lost focus)
Here is the driver code for calling this Dialog.
import tkinter as tk
def createModal():
Modal(root)
root = tk.Tk()
button = tk.Button(root, text="Create Modal", command=createModal)
button.pack(padx = 50, pady = 40)
root.mainloop()
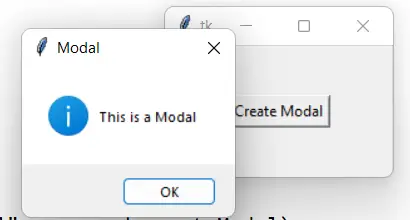
We can also further customize the Modal by making the label text and title as parameters which can be passed in.
class Modal(tk.Toplevel):
def __init__(self, master, title, message, *args):
tk.Toplevel.__init__(self, master, *args)
self.title(title)
tk.Label(self, text = message).pack(padx=30, pady=20)
self.grab_set()
class Modal(tk.Toplevel):
def __init__(self, master, title, message, *args):
tk.Toplevel.__init__(self, master, *args)
self.title(title)
tk.Label(self, text = message).pack(padx=30, pady=20)
tk.Button(self, text = "Close", command=self.destroy)
self.grab_set()
self.overrideredirect(True)
Another cool thing that we can do is remove the “window bar” that at the top of the Modal (with the minimize, maximize and close buttons). This gives it a more of a “dialog” feel and might be better in some situations.
We’ll have to provide a way of closing the Dialog in this case though, so we’ll add a button to destroy the window.
Note: If you are having trouble with Modal window not appearing in the center of the screen, refer to this tutorial on center-ing windows.
Modals with Tkinter MessageBox
Here we will create a Modal using a submodule from Tkinter called “messagebox”. This library has several functions which create pre-made Modal Dialogs for us. We can customize certain things, such as the title and the text that appears.
import tkinter as tk
from tkinter import messagebox
def createModal():
dlg = messagebox.showinfo("Modal", "This is a Modal")
root = tk.Tk()
button = tk.Button(root, text="Create Modal", command=createModal)
button.pack(padx = 50, pady = 40)
root.mainloop()
If you are looking for a simple solution, messagebox is great for most cases. If you want more customization somewhere down the line, you can switch to the Custom approach from before.
This marks the end of the How to create a Modal dialog box in Tkinter? Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions about the tutorial content can be asked in the comments section below.