Tkinter is a great Python library used to create GUI programs. Using Tkinter, you can make a wide variety of different desktop applications, which you could then distribute to clients and customers. But how would you go about distributing your Tkinter application? That’s where Pyinstaller comes in, as it has the ability to convert Tkinter and Python programs into a distributable Exe.
The Tkinter Program
So here’s our Tkinter program, which we will be converting to a Pyinstaller EXE.
import tkinter as tk
import os, sys
from PIL import Image, ImageTk
class Window:
def __init__(self, master):
self.img = Image.open("M_Cat_Loaf.jpeg")
self.img = self.img.resize((320, 300), Image.ANTIALIAS)
self.img = ImageTk.PhotoImage(self.img)
label = tk.Label(master, image = self.img)
label.pack(expand = True, fill = tk.BOTH)
root = tk.Tk()
window = Window(root)
root.mainloop()
The purpose of this program is simple. It loads an image, and displays that image in a GUI window. Our goal is to successfully compile this program into an EXE using Pyinstaller.
Converting our Tkinter Program to Pyinstaller Exe
So how can we go about doing this? Well, first you need to setup Pyinstaller. You can do this through the terminal or command prompt using pip or any equivalent method.
pip install pyinstaller
Next, you to evaluate your program and what special pyinstaller options you may have to use. If you run the following command directly, there will be several issues.
pyinstaller mypythonfile.py
Pyinstaller command are used in the command prompt, or in a terminal. Make sure you either have your Python Installation included in “PATH” otherwise you will need to run this command in the same folder as your Python installation.
While this command would work for a regular Python script, it will cause several problems for our Tkinter Program. Firstly, our Tkinter GUI program is Window Based, not Console Based. Pyinstaller by default pops open a console window whenever the exe is run.
That’s obviously going to be a bit jarring, because you will not be using the Console Window when you have your own GUI with Tkinter. To disable this console window, we need to add the following option.
pyinstaller --noconsole mypythonfile.py
We still aren’t done though. Our Tkinter program uses an image, which is fairly common for GUI programs. To include this, we need to use the --add-data
option.
pyinstaller --noconsole --add-data "C:/Desktop/M_Cat_Loaf.jpeg;." mypythonfile.py
Now our command is valid, and will generate the required exe file! The above command will generate an entire directory, in which there will be many library related files, as well as the application exe.
The Output
Here’s a (partial) screenshot. (The name of my python file was PILImageTk.py, so the same name was given to the exe)
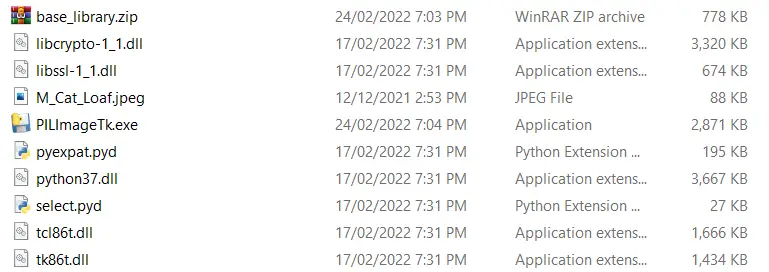
Want to learn more about Pyinstaller and it’s various features? Check our dedicated tutorial on it for more!
Having trouble with Pyinstaller? Check out this tutorial on common problems with Pyinstaller and how to resolve them.
This marks the end of the “Using Tkinter with Python Pyinstaller” Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.