In this Tkinter Tutorial we will explore the ttk ProgressBar widget, and how it can be used to create loading screens or show the progress of a current task(s). As always, there is a set of useful options and methods which we will discuss in the examples below.
A complete list of widget-specific options can be found at the bottom of the tutorial.
ttk ProgressBar – Syntax
The syntax for the ProgressBar widget.
ttk.Progressbar(parent, orient, length, mode)
Parent: The container in which the ProgressBar is to be placed, such as root or a Tkinter frame.
Orient: Defines the orientation of the ProgressBar, which can be either vertical of horizontal.
Length: Defines the width of the ProgressBar by taking in an integer value.
Mode: There are two options for this parameter, determinate and indeterminate.
In indeterminate mode, there is a flashing light that moves back and forth, throughout the progressbar. This is used when you want to show loading progress, but don’t know how long it will take, or how much progress has been made.
Determinate mode is your regular ProgressBar, that starts from 0, and slowly fills up the whole bar with a green color to show current progress. (This is the default)
ProgressBar Example# 1
As this is our first example, let’s focus on creating a simple progress bar with some basic functions. The below code creates a progressbar with three buttons which are linked to three different functions.
The first function increments the “value” or “progress” in the progressbar by 20. (By default, the maximum value in a progressbar is 100). This is done with the step() function which takes an integer value to change progress amount. (Default increment of the step()
is 1.0)
The second function decrements the “value” or “progress” in the progressbar by 20.
The third function prints out the current progress level in the progressbar.
import tkinter as tk
from tkinter import ttk
class Window:
def __init__(self, master):
self.master = master
self.frame= ttk.Frame(self.master)
self.progressBar= ttk.Progressbar(self.frame, mode='determinate')
self.progressBar.pack(padx = 10, pady = 10)
self.button= ttk.Button(self.frame, text= "Increase",
command= self.increment)
self.button.pack(padx = 10, pady = 10, side = tk.LEFT)
self.button= ttk.Button(self.frame, text= "Decrease",
command= self.decrement)
self.button.pack(padx = 10, pady = 10, side = tk.LEFT)
self.button= ttk.Button(self.frame, text= "Display",
command= self.display)
self.button.pack(padx = 10, pady = 10, side = tk.LEFT)
self.frame.pack(padx = 5, pady = 5)
def increment(self):
self.progressBar.step(20)
def decrement(self):
self.progressBar.step(-20)
def display(self):
print(self.progressBar["value"])
root = tk.Tk()
window = Window(root)
root.mainloop()
The output:
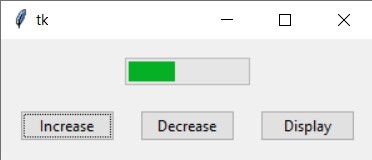
ProgressBar Example# 2
In this example we will create a progressbar in “indeterminate” mode. Luckily, this just requires a single change to the “mode” in the ProgressBar Class Constructor.
There are some other changes we need to make too, as it doesn’t make sense to have “increment” and “decrement” with “indeterminate” mode. Instead we have “start” and “stop”. (Both of these functions can be used on determinate mode as well)
The start button will begin an autoincrement mode, that calls the step() function every 50 milliseconds. You can change the interval duration by passing a value in the start function, like progressbar.start(10)
.
The stop button merely “stops” the auto-increment mode, and resets it back to zero.
import tkinter as tk
from tkinter import ttk
class Window:
def __init__(self, master):
self.master = master
self.frame= ttk.Frame(self.master)
self.progressBar= ttk.Progressbar(self.frame, mode='indeterminate')
self.progressBar.pack(padx = 10, pady = 10)
self.button= ttk.Button(self.frame, text= "Start",
command= self.start)
self.button.pack(padx = 10, pady = 10, side = tk.LEFT)
self.button= ttk.Button(self.frame, text= "Stop",
command= self.stop)
self.button.pack(padx = 10, pady = 10, side = tk.LEFT)
self.button= ttk.Button(self.frame, text= "Display",
command= self.display)
self.button.pack(padx = 10, pady = 10, side = tk.LEFT)
self.frame.pack(padx = 5, pady = 5)
def start(self):
self.progressBar.start(interval = 10)
def stop(self):
self.progressBar.stop()
def display(self):
print(self.progressBar["value"])
root = tk.Tk()
window = Window(root)
root.mainloop()
The output:
ProgressBar Example# 3
In this example we will attempt to cover as many other ProgressBar options and settings as possible. The syntax is pretty simple, so following it should be a piece of cake.
The main features found in this example:
- Makes use of lambda to avoid having to write a separate function.
- Changes the orientation of the ProgressBar
- Customizes the stop command, so it just pauses the progressbar, instead of resetting it.
- Made a separate button for Reset.
- Changed the length and maximum value for the progressbar. (Length is just the width, and has nothing to do with the maximum possible value of the progressbar)
import tkinter as tk
from tkinter import ttk
class Window:
def __init__(self, master):
self.master = master
self.frame= ttk.Frame(self.master)
self.progressBar= ttk.Progressbar(self.frame, mode='determinate',
maximum=200, length=200, orient= tk.VERTICAL)
self.progressBar.pack(padx = 10, pady = 10, side = tk.RIGHT)
self.button= ttk.Button(self.frame, text= "Reset",
command= self.reset)
self.button.pack(padx = 10, pady = 10, side = tk.BOTTOM)
self.button= ttk.Button(self.frame, text= "Print",
command= self.display)
self.button.pack(padx = 10, pady = 10, side = tk.BOTTOM)
self.button= ttk.Button(self.frame, text= "Increase",
command= self.increment)
self.button.pack(padx = 10, pady = 10, side = tk.BOTTOM)
self.button= ttk.Button(self.frame, text= "Stop",
command= lambda: self.stop())
self.button.pack(padx = 10, pady = 10, side = tk.BOTTOM)
self.button= ttk.Button(self.frame, text= "Start",
command= lambda: self.progressBar.start(10))
self.button.pack(padx = 10, pady = 10, side = tk.BOTTOM)
self.frame.pack(padx = 5, pady = 5)
def increment(self):
self.progressBar.step(20)
def display(self):
print(self.progressBar["value"])
def stop(self):
val = self.progressBar["value"]
self.progressBar.stop()
self.progressBar["value"] = val
def reset(self):
self.progressBar["value"] = 0
root = tk.Tk()
window = Window(root)
root.mainloop()
The output of the above code: (Run it yourself for the best experience)
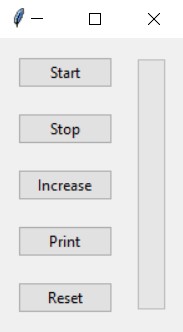
ttk ProgressBar Options
A list of options for the Tkinter ttk ProgressBar.
Option | Description |
---|---|
orient | Either “horizontal” or “vertical”. Specifies orientation. |
length | The width of the progress bar. |
mode | Either “determinate” or “indeterminate”. (Described in examples above) |
maximum | A number specifying the maximum value possible value (Default is 100). |
value | The current value of the progress bar. In indeterminate mode, the value accumulates as it cycles back and forth. So if maximum is 100, and 2 cycles were completed, current value will show as 200. |
variable | A name which is linked to the option value. If specified, the value of the progress bar is automatically set to the value of this name whenever the latter is modified. |
phase | Read-only option. The widget periodically increments the value of this option whenever its value is greater than 0 and, in determinate mode, less than maximum. This option may be used by the current theme to provide additional animation effects. |
This marks the end of the Tkinter ttk ProgressBar Widget Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.