In this tutorial we will explore how to perform a Union operation between two Polygons in Shapely. The union operation is commonly used to combine intersecting Polygons into one combined Polygon by dissolving certain edges and combining common points.
What happens to non-intersecting Polygons though? Read further in the tutorial to find out!
How to perform Union on Polygons
Shapely has an old deprecated function for performing union, called cascaded_union()
. If you see anyone using this, you should know that its old and outdated. Don’t use it anymore.
Shapely has introduced a newer and better version called unary_union()
which we will be using in this tutorial.
All you need to do is pass in a list of Polygons (can be any number of Polygons) to this function, and it will return a brand-new combined Polygon.
from shapely.geometry import Polygon
from shapely.ops import unary_union
poly_1 = Polygon([(20, 20), (60, 20), (60, 40), (20, 40)])
poly_2 = Polygon([(40, 30), (40, 70), (80, 70), (80, 30)])
new_poly = unary_union([poly_1, poly_2])
The above code performs a union operation, and saves the result in new_poly
. But how do we know that our union operation was carried out successfully?
There are two ways of doing this. We can either print out the Polygon and manually double check the coordinates as shown below.
print(new_poly)
POLYGON ((60 20, 20 20, 20 40, 40 40, 40 70, 80 70, 80 30, 60 30, 60 20))
Or, we can go ahead and use matplotlib to plot it.
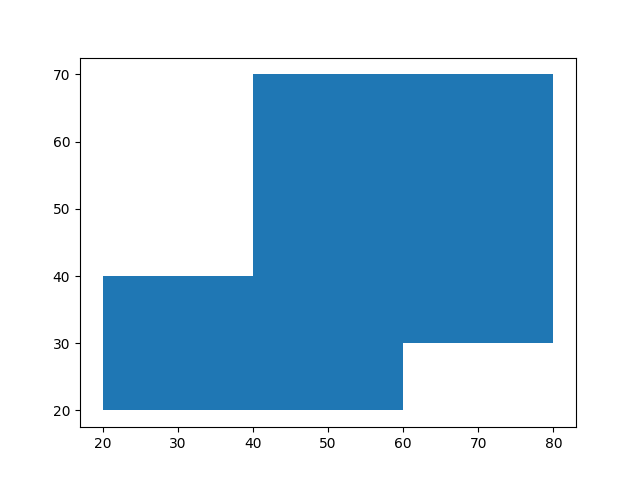
Want to learn how to do this too? Refer to our Matplotlib + Shapely Visualization tutorial.
Dealing with MutliPolygons
There is another possible outcome that you may run into while performing on two (or more) Polygons. If the Polygons you passed to unary_union()
do not intersect at all, then it will return a Multi-polygon. You can double check this by using the type
attribute which returns a string value (e.g, “Polygon” or “MultiPolygon”.
print(new_poly.type)
A MultiPolygon is actually just a collection of individual polygons. We can actually access each Polygon inside it by iterating over it as shown below.
from shapely.geometry import Polygon
from shapely.ops import unary_union
poly_1 = Polygon([(20, 20), (60, 20), (60, 40), (20, 40)])
poly_2 = Polygon([(60, 50), (60, 70), (80, 70), (80, 50)])
new_poly = unary_union([poly_1, poly_2])
print("Type: ", new_poly.type)
for poly in new_poly.geoms:
print(poly)
Type: MultiPolygon
POLYGON ((20 40, 60 40, 60 20, 20 20, 20 40))
POLYGON ((60 70, 80 70, 80 50, 60 50, 60 70))
There aren’t many operations you can directly perform on multi-polygons, so you typically have to use the above technique and then perform that operation on each individual polygon (like extracting coordinates or plotting them).
This marks the end of the Union of Polygons in Shapely Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.