In this “Tkinter vs wxPython” article we will compare two popular GUI libraries in Python, to determine the winner. Through the article, we will attempt to highlight the advantages and disadvantages of using either library, and share some code snippets to help you decide which one you should be learning.
Table of Contents
- Tkinter
- wxPython
- Communities
- wxPython vs Tkinter (Code Comparison)
- Conclusion
Tkinter
As the standard de-facto GUI Library for Python, let’s talk about Tkinter first.
Advantages
Tkinter is one of the oldest GUI libraries around, allowing it to amass a massive userbase eventually leading it to be added in the Standard Python Library. This gives it the benefit of not requiring any additional installation or downloading of packages. It’s also a very mature and stable library, so you are not likely to run into any issues.
Tkinter’s main plus points can be summarized in a single sentence. It’s simple, fast and easy to learn. These three points are the main reason behind Tkinter’s popularity, despite the a few disadvantages that we’ll talk about later on.
A few other less talked about advantages, but important ones none-the-less, are Tkinter’s (Event) Binding system and it’s Layout Management. The Binding System is super flexible and easy to integrate, allowing you to do things like triggering functions upon mouse hover or a keypress.
Likewise, the Layout system in Tkinter is pretty intuitive and easy to setup using one of the three layout managers, pack(), place() or grid(). You will likely find Tkinter’s Layout and Binding System to be more powerful and easier to use than wxPython.
Disadvantages
It undoubtedly has a very “classic” look to it and can be used to create professional applications in the right hands. However, it’s out of the box look is outmatched by almost every other GUI library, such ax wxPython and PyQt.
Another shortcoming in Tkinter is it’s widgets. While they are pretty simple and intuitive to use, they have a noticeably outdated look to them. Furthermore, other libraries like PyQt and wxPython have more powerful and advanced widgets, such as the PyQt video player. (Tkinter matches up pretty well when it comes to the base widgets, but not when it comes to the more advanced stuff)
ttk extension
If not for the ttk submodule, Tkinter’s disadvantages would likely convince some to switch over to another GUI library. However ttk helps to mitigate this, by introducing themes and new styling options, as well as an updated look for many widgets.
Here’s what some of the default tkinter widgets look like.
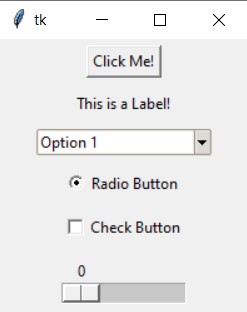
And here’s the same thing, but with ttk versions of the widgets instead.
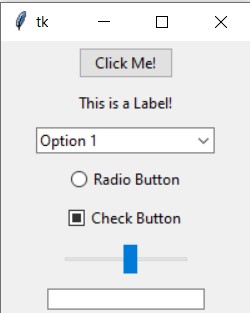
As you can see, the lower one looks more neater and modern. There are other special effects like when you hover over the button (which gives it a blue highlight), that is not present in the default Tkinter. You can even change and customize the theme further, however you like (and it’s easy to do as well!)
Also, please do note that ttk is not a separate library or package that needs to be downloaded separately. It’s included within the tkinter library, and can easily be imported. You can learn more about ttk and it’s widgets in this ttk Tutorial Series!
wxPython
And for wxPython, which is a Python extension of the wxWidgets Library (a cross-platform library)
Advantages
wxPython’s main advantages and it’s selling point is in it’s large variety of feature rich widgets, and it’s good design and look. These are also it’s main advantages over the Tkinter library, which looks a bit more dated. Another plus point is that wxPython looks great across all platforms right out of the box, and doesn’t really require any custom tinkering.
It may have a steeper learning curve than Tkinter, but once you grasp it’s workings, you will have access to more features and functionalities than you would in Tkinter.
Those of you interested in a more native windows look will appreciate wxPython, as it resembles the native Windows GUI.
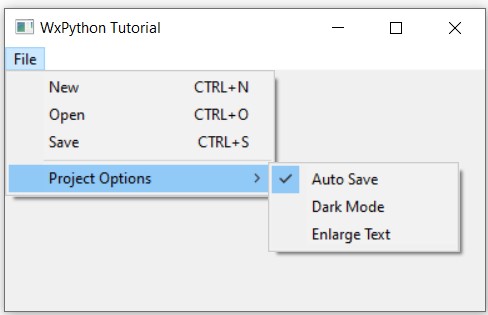
The Menu widget is a good example, where wxPython comes built-in with support for Menu Icons, key-board shortcuts and handy menu-item widgets like the check-button-menu which you can see in the image above.
While we are on the topic, I would consider the Menu widget in wxPython to look better than its Tkinter counterpart (without ttk).
Disadvantages
To start off, the very thing I want to say is that wxPython requires a separate download (luckily the process is simple using pip). This is something that may matter when you are distributing your applications around to other users. Otherwise this is an almost negligible issue, especially if it’s just for personal issue.
Another “con” for wxPython is that it’s a tad bit slower than Tkinter, though this is a bit hard to measure outside of the initial loading for the window to display.
Another slight concern may be that wxPython is still under active-development, though this also works in it’s favor in a sense. It hasn’t reached the level of stability and maturity that Tkinter has, but it’s active development has the potential to bring in some new features that could help tip the scales in wxPython’s favor.
We’ve already discussed a few areas where Tkinter beats out wxPython in the Tkinter section, so lets not bring that up again. However, if we look at wxPython from a neutral standpoint, there aren’t really any significant cons (aside from the speed issue).
Tkinter Community vs wxPython Community
Let’s talk about the community briefly, before we move onto the Code Comparison. Community is pretty important, and determines the lifespan of a library, as well as the amount of effort required to learn the library.
One slight issue I had while learning wxPython was the lack of online tutorials and resources outside of the documentation. Many wxPython tutorials that appear on the first page of google are a decade old, and not updated to conform with the latest wxPython version (Phoenix). This is also partly because wxPython is still under active development. The documentation is decent though, so that does make up for it.
(We recently released a whole Video series on YouTube for wxPython’s latest version to help out with this problem. Give it a watch if you are interested!)
Tkinter on the other hand, has a ton of community support, and there are dozens of up-to-date guides that you can find online. Tkinter’s popularity is evident by the fact that it’s in the Python Standard Library.
In short, if we compare the Tkinter Community vs wxPython Community, Tkinter has the edge.
wxPython vs Tkinter – Code Comparison
Just for the sake of it, let’s take a look at some code from both libraries, just to so you can get a feel for the syntax and what the output looks like.
It’s just a simple example where we create a single button linked to a function. The examples should show you the basic code required to setup a window and a simple widget in wxPython/Tkinter.
First, we have Tkinter.
import tkinter as tk
class Window:
def __init__(self, master):
self.master = master
frame = tk.Frame(self.master)
button = tk.Button(frame, text = "Close Window", command = self.quit)
button.pack(pady = 30)
frame.pack()
def quit(self):
self.master.destroy()
root = tk.Tk()
root.geometry('200x150')
window = Window(root)
root.mainloop()
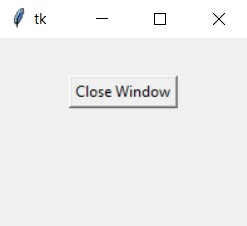
Here’s the wxPython version.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
panel = wx.Panel(self)
closeButton = wx.Button(panel, label = "Close", pos = (50,50), size = (100,30))
closeButton.Bind(wx.EVT_BUTTON, self.closeWindow)
self.Centre()
self.Show()
def closeWindow(self, e):
wx.CallAfter(self.Close)
app = wx.App()
window = Window("WxPython Button Tutorial")
app.MainLoop()
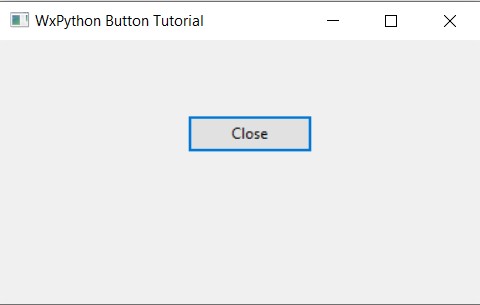
(The default wxPython window size is rather big, hence why it looks that way, don’t be alarmed)
You can’t really conclude much from these examples or images, but it should give a very rough idea on what to expect.
wxPython vs Tkinter – Conclusion
If I could somehow summarize in a sentence, I would be tempted to say that Tkinter is better designed and a little easier to use, but wxPython is more feature-rich, powerful and looks a little bit nicer. This I personally think is a statement that accurately captures the main difference between the two.
Just remember, both are great libraries and both are used to make powerful GUI applications. In the end, what matters more is your own skill in utilizing the libraries features. A poorly developed Tkinter application is far worse than a well developed wxPython application, and vice-versa.
If you are looking for a third option, I would recommend PyQt, which is the go-to choice for many Python users when it comes to building powerful and good-looking GUI. You can learn more about it from our PyQt Tutorial Series!
Hopefully we were able to resolve the wxPython vs Tkinter debate for you, and helped you make up your mind.
Let us know down in the comments section below which one you prefer, and why? Do you think wxPython is the best GUI library, or Tkinter? Or maybe some other library like PyQt?
This marks the end of the Tkinter vs wxPython comparison. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.