In this wxPython Tutorial, we will demonstrate how to use the StaticText Widget, alongside it’s various styles, features and functions. A complete list of options will be included here, alongside several code examples for your convenience.
wxPython StaticText Syntax
The syntax required to create a StaticText widget.
text = wx.StaticText(parent, id, label, pos, size, style)
parent
: This is the Widget to which it is parented, such as a Panel.id
: Widget ID. Default value is ID_ANY, which gives it the next available ID.label:
The Text which you want to display on the Widget.pos:
A tuple containing the coordinates of where the topleft corner of the StaticText Widget should begin from.size:
A tuple which defines the dimensions of the area occupied by the Text.style:
Used for styling the Text (such as Alignment)
StaticText Styles
A list of Styles available for the StaticText Widget.
Style Name | Description |
---|---|
wx.ALIGN_LEFT | Aligns the text to the Left |
wx.ALIGN_RIGHT | Aligns the text to the Right |
wx.ALIGN_CENTRE_HORIZONTAL | Center Aligns the Text (Horizontally) |
wx.ST_NO_AUTORESIZE | Does not Auto-Resize the text if it is changed using SetLabel(). Useful for if you have used certain alignment options. |
wx.ST_ELLIPSIZE_START | If the Text exceeds the size of the widget, this style replaces the beginning of the Text with Ellipsis (…). |
wx.ST_ELLIPSIZE_MIDDLE | If the Text exceeds the size of the widget, this style replaces the middle of the Text with Ellipsis (…). |
wx.ST_ELLIPSIZE_END | If the Text exceeds the size of the widget, this style replaces the end of the Text with Ellipsis (…). |
StaticText Example
Below is an example of a simple GUI window that is displaying some text.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title, size = (420, 300))
self.panel = wx.Panel(self)
wx.StaticLine(self.panel, pos=(20, 240), size=(360,1), style = wx.HORIZONTAL)
content1 = '''Lorem ipsum dolor sit amet, consectetur adipiscing elit.
Vestibulum facilisis consequat tellus quis consectetur'''
content2 = '''Quisque pretium venenatis interdum'''
text1 = wx.StaticText(self.panel, label = content1, pos = (60, 100))
text2 = wx.StaticText(self.panel, label = content2, pos = (60, 160))
wx.StaticLine(self.panel, pos=(20, 20), size=(360,1), style = wx.LI_HORIZONTAL)
self.Centre()
self.Show()
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
We have used multi-line strings to store the text that we wish to display in the StaticText widget. We have also used some StaticLine widgets
The output:
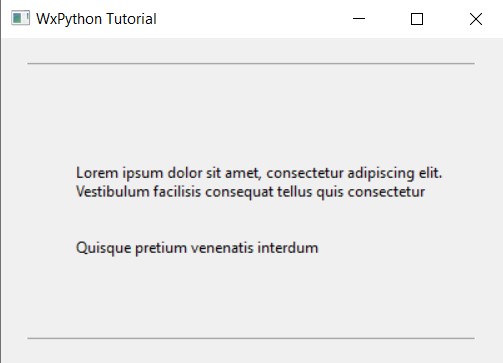
Changing Text with SetLabel()
You can change the text displayed on the StaticText Widget, even after it has been defined using SetLabel()
. This function takes a string which will replace the currently displayed string.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title, size = (420, 300))
self.panel = wx.Panel(self)
content1 = '''Lorem ipsum dolor sit amet, consectetur adipiscing elit.
Vestibulum facilisis consequat tellus quis consectetur'''
content2 = '''Quisque pretium venenatis interdum'''
self.text1 = wx.StaticText(self.panel, label = content1, pos = (40,100), size = (340, 40))
self.text2 = wx.StaticText(self.panel, label = content2, pos = (40,160), size = (340, 30))
mybutton = wx.Button(self.panel, label = "Change Text", pos = (100, 200))
mybutton.Bind(wx.EVT_BUTTON, self.ChangeText)
self.Centre()
self.Show()
def ChangeText(self, e):
self.text1.SetLabel("REPLACED WITH RANDOM TEXT")
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
Clicking on the button will change the prior text to:
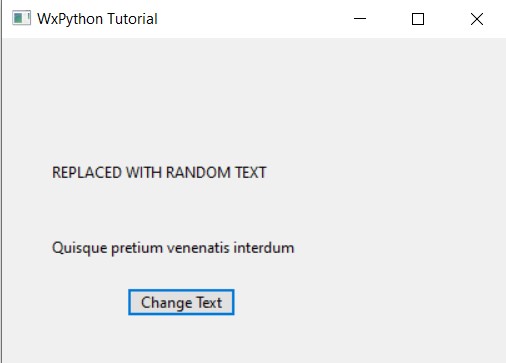
Likewise, you can use GetLabel()
to get a string containing the text currently displayed on the StaticText Widget.
Changing StaticText Color
Changing the Text Color on the StaticText widget can easily be done using SetForegroundColour()
which takes as parameter a string and colors the text accordingly.
text1.SetForegroundColour("blue")
text2.SetForegroundColour("red")
text1.SetBackgroundColour("yellow")
text2.SetBackgroundColour("green")
Similarly you can use SetBackgroundColour()
which sets the background color. Any area occupied by the StaticText widget, even if there is no text there, will be colored. (You’ll notice this when we use the size
option later)
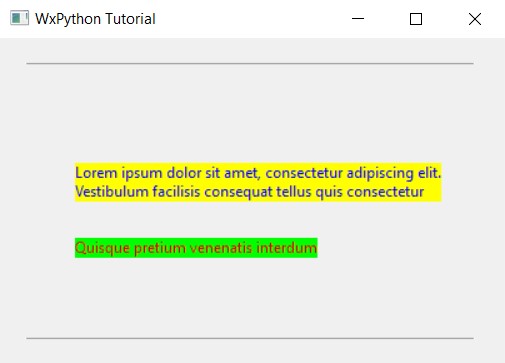
As an alternative, and a more flexible option is to use Hexadecimal codes as shown below. This allows you to pick from a wide variety of colors.
text1.SetForegroundColour("#0000FF")
text2.SetForegroundColour("#FF0000")
The above code is the exact same as the one from earlier, but with hex codes instead.
Adding Styles
In this section we’ll use the size
and style
(Alignment) options for the wxPython StaticLine widget. By default, the StaticText widget will adjust it’s size to match the content inside of it (This can be prevented with wx.ST_NO_AUTORESIZE
).
You won’t really notice the style changes until you’ve set a manual size for the StaticText Widget. This is because there is no room for alignment under the default settings.
The below code set’s manual sizes for both StaticText Widgets and applies some Alignment Styles.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title, size = (420, 300))
self.panel = wx.Panel(self)
wx.StaticLine(self.panel, pos=(20, 240), size=(360,1), style = wx.HORIZONTAL)
content1 = '''Lorem ipsum dolor sit amet, consectetur adipiscing elit.
Vestibulum facilisis consequat tellus quis consectetur'''
content2 = '''Quisque pretium venenatis interdum'''
text1 = wx.StaticText(self.panel, label = content1, pos = (40,100),
size = (340, 40), style = wx.ALIGN_CENTRE_HORIZONTAL)
text2 = wx.StaticText(self.panel, label = content2, pos = (40,160),
size = (340, 30), style = wx.ALIGN_RIGHT)
text1.SetForegroundColour("#0000FF")
text2.SetForegroundColour("#FF0000")
text1.SetBackgroundColour("yellow")
text2.SetBackgroundColour("green")
wx.StaticLine(self.panel, pos=(20, 20), size=(360,1), style = wx.LI_HORIZONTAL)
self.Centre()
self.Show()
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
The output:
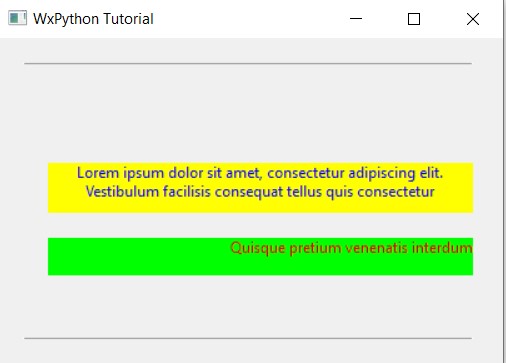
This marks the end of the wxPython StaticText Widget Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.