This article covers the JavaFX RadioButton widget.
RadioButtons are a common GUI element for taking User input. Along with Checkboxes, they display to the User a set of options in form of a selectable circle/box. Unlike CheckBoxes however, only one radiobutton amongst a group can be selected.
You will find a complete list of methods available for the JavaFX Radiobutton at the bottom of this page.
JavaFX RadioButton Example
We’ll be creating two radio buttons in the example below. The reason being that RadioButtons are rarely used alone. Besides, unless we create multiple radio-buttons we won’t be able to showcase the Grouping ability of radio buttons. This is important because as per the role of the radiobutton, only one radiobutton in a group should be able to be selected at a given time.
We’ll use the RadioButton and ToggleGroup classes to make a radiobutton and togglegroup respectively. Finally, we’ll add each radiobutton to the togglegroup individually using the setToggleGroup()
method.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.RadioButton;
import javafx.scene.layout.VBox;
import javafx.scene.control.ToggleGroup;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
VBox layout = new VBox();
Scene scene = new Scene(layout, 250, 150);
RadioButton radio1 = new RadioButton("Option 1");
RadioButton radio2 = new RadioButton("Option 2");
ToggleGroup radioGroup = new ToggleGroup();
radio1.setToggleGroup(radioGroup);
radio2.setToggleGroup(radioGroup);
layout.getChildren().addAll(radio1, radio2);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
The GUI output of the above code is shown below.
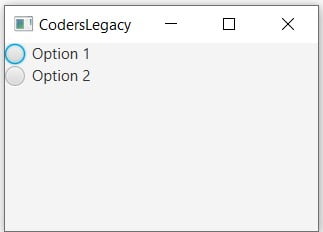
Also keep in mind, you cannot “de-select” a radio button. You can only remove the selection from it by clicking on another radiobutton from the same group.
Adding radio-buttons into a group is absolutely essential. Else they will exist as individual radio-buttons with no link to each other. The Group system ensures that only one can be selected, not more than that. You can of course, have multiple groups in your GUI.
Retrieving RadioButton Values
We’ll be creating a button widget and linking it to the methods we’ll be discussing below. The button is like our “trigger” which when pressed, activates our main block of code for value retrieval.
The getSelectedToggle()
function is meant to be used on togglegroups. It will return the currently selected radiobutton. It does not return a simple value, rather it returns a sort of object. Hence, we first must convert it to a RadioButton, then we can use the regular methods to find out more about the radiobutton.
package application;
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.RadioButton;
import javafx.scene.control.ToggleGroup;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
VBox layout = new VBox();
Scene scene = new Scene(layout, 250, 150);
RadioButton radio1 = new RadioButton("Option 1");
RadioButton radio2 = new RadioButton("Option 2");
ToggleGroup radioGroup = new ToggleGroup();
radio1.setToggleGroup(radioGroup);
radio2.setToggleGroup(radioGroup);
Button button = new Button("Submit");
button.setOnAction(e -> {
RadioButton temp = (RadioButton) radioGroup.getSelectedToggle();
System.out.println(temp.getText());
});
layout.getChildren().addAll(radio1, radio2, button);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
An Image of the GUI output taken next to the Console output on the Eclipse IDE. First we selected Option 2 and pressed the submit button. Then we selected Option 1 and pressed the button again.
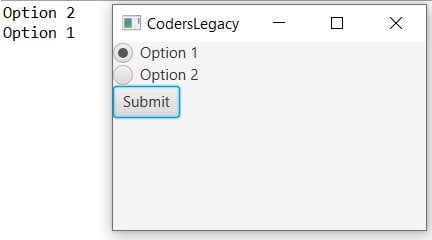
RadioButton Methods
This is a compilation of many useful methods that can be used with the JavaFX RadioButton widget.
Method | Description |
---|---|
getText() | Returns the text value of the radiobutton. |
isSelected() | Returns whether the radiobutton is selected or not. |
setSelected(bool) | Selects or Deselects the radiobutton depending on the boolean value passed. |
setToggleGroup(group) | Adds the radiobutton to the toggle group passed into it’s parameters. |
This marks the end of the JavaFX RadioButton article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.