This article covers the JavaFX Label widget.
The JavaFX Label widget is one of the simplest widgets you’ll see in a GUI program. It’s used simply to display text onto the screen. Alternatively, it can also be used display images.
The Label widget is imported with the following name: javafx.scene.control.Label
.
JavaFX Label Example
There are only three label related lines in the below code. First is the Label import. Second is the creation of the label using the Label Class. Finally, we add the label into the layout we created.
Whatever text you want to be displayed, make sure to pass it into the Label Class while creating the label.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
StackPane layout = new StackPane();
Scene scene = new Scene(layout, 300, 300);
Label label = new Label("JavaFX Label Demonstration");
layout.getChildren().addAll(label);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
The output of the above code:
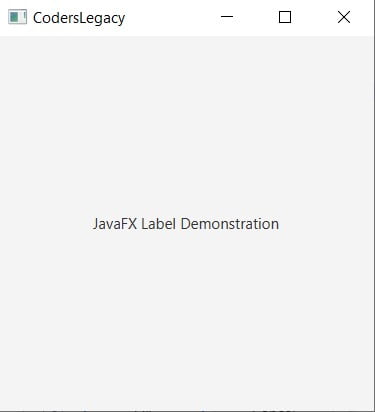
If you want to learn how to display images in JavaFX using Labels, head over to our JavaFX ImageView tutorial.
Label Font
With the help of the setFont()
you can change the font-family and font size of the Text on the label.
Creating a font object is simple. You just need to pass two things into the Font class, a font family and size. Be careful though, not all font families are going to be supported by JavaFX or on the system you plan to run your program.
Remember to include that extra Font import!
import javafx.application.Application;
...
...
import javafx.scene.text.Font;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
StackPane layout = new StackPane();
Scene scene = new Scene(layout, 300, 300);
Label label = new Label("JavaFX Label Demonstration");
label.setFont(new Font("Arial", 20));
layout.getChildren().addAll(label);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
The output of the above code. Notice how different the font is compared to the previous one.
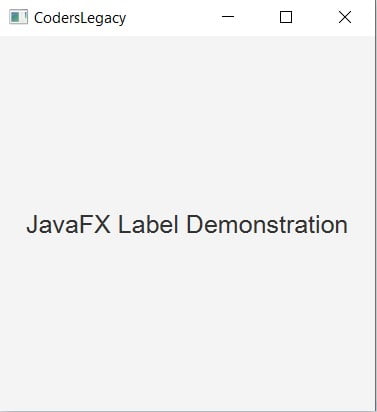
Here is a small list of different Fonts that we personally tried and tested.
- Arial
- Calibri
- Verdana
- Helvetica
- Times New Roman
- Garamond
- Comic Sans MS
- Candara
In the event that a Font style you have tried doesn’t work, it will not throw any error, instead reverting a “Default font” instead.
Changing the Label Text
Most people at some point need a way to be able to change the text of the Label, either by the user or the program during execution. This is a short tutorial on how to do so.
In short, we create a JavaFX button and link it’s “click” action to the setText()
method for the label.
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
VBox layout = new VBox();
Scene scene = new Scene(layout, 300, 300);
Label label = new Label("JavaFX Label Demonstration");
Button button = new Button("Press me");
button.setOnAction(e -> label.setText("Label Text Change Demonstration"));
layout.getChildren().addAll(button, label);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
A short GIF demonstrating this ability:
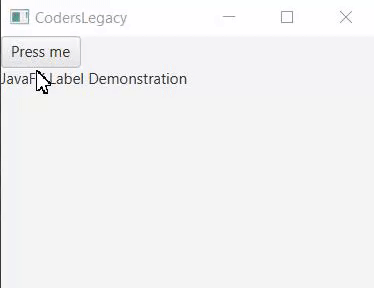
JavaFX Label Methods
A list of important and useful methods for the Label widget.
Method | Description |
---|---|
setFont() | Takes a Font object as input, changing the Font of the displayed text accordingly. |
setText() | Used to set the current (displayed) text. |
getText() | Returns the current text value. |
setAlignment(pos) | Used to set the text alignment of the text in the widget. Full list of positions available here. |
setMaxWidth(n) | Sets the maximum possible value of the width. Measured in pixels. |
setMinWidth(n) | Sets the minimum possible value of the width. Measured in pixels. |
This marks the end of the JavaFX Label article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.