In this JavaFX tutorial we will explore how to change the Default Background Color of our “Scene”. We will discuss several techniques we can use this to do this, and how to apply various color styles to our backgrounds in JavaFX.
Setting Background Color on Scene
Setting the background Color of a scene is actually very simple. All we need to do is pass a fourth parameter to the Scene Class, which contains our Color information. There are multiple ways of defining colors as we will soon see.
The easiest way is to use one of the pre-built colors provided by JavaFX. e.g, Color.BLUE, Color.RED, Color.GREEN etc.
Scene scene = new Scene(layout, 300, 300, Color.BLUE);
Here is the full running code, which includes all the necessary imports. Don’t forget the extra import for the Color class.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
public class Main extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
StackPane layout = new StackPane();
Scene scene = new Scene(layout, 300, 300, Color.BLUE);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
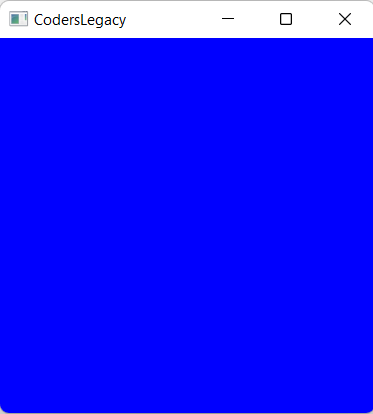
Alternate Methods
Alternatively you can call the setFill()
method on the scene object to change its color.
scene.setFill(Color.BLUE);
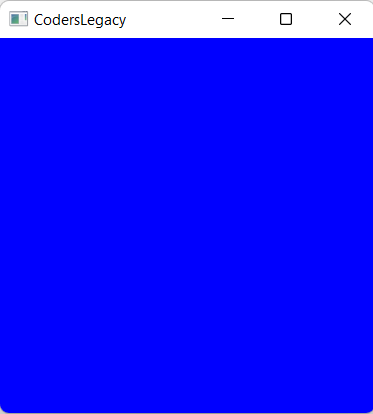
Instead of using the pre-built Colors provided by JavaFX, we can instead use “hex color codes” for a greater variety. Below is a small example of how we can do this.
scene.setFill(Color.web("#Ae1253"));
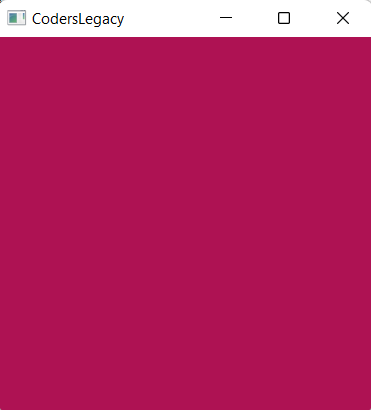
Setting Background Color on Layouts
We don’t directly have to set the color of the Scene itself. Instead, we can place a layout inside the scene, and then we fill in the color for the layout, which provides the exact same effect as before.
Here is a small example where instead of setting the Scene color, we set the Layout color instead.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
public class Main extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
StackPane layout = new StackPane();
Scene scene = new Scene(layout, 300, 300);
layout.setStyle("-fx-background-color: blue");
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
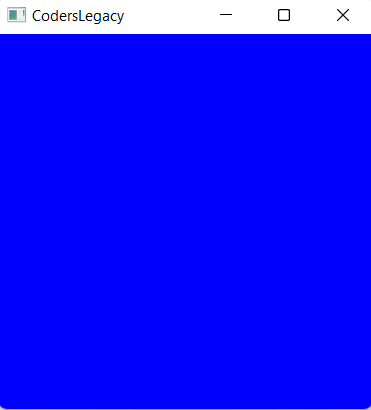
Another benefit of this technique, is that we can have multiple layouts inside a Scene. This allows us to introduce multiple colors into our window.
Here is a small example featuring two layouts, with one of them being nested in the other, to create a Multi-color effect.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
public class Main extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primary) throws Exception {
Button button = new Button("Hello");
VBox layout = new VBox(10);
VBox sublayout = new VBox(10, button);
Scene scene = new Scene(layout, 300, 300);
layout.getChildren().addAll(sublayout);
sublayout.setStyle("-fx-background-color: blue");
layout.setStyle("-fx-background-color: red");
primary.setTitle("Layouts Color Tutorial");
primary.setScene(scene);
primary.show();
}
}
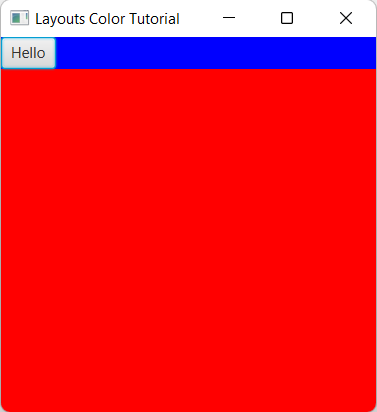
This marks the end of the JavaFX Background Color Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.
Good and useful explanation.