Every GUI Window needs a way of taking input from the User. In PyQt6, the most common (and easiest) way of taking input is by using the QLineEdit widget. It offers you a single Input Box, where you can input a single line of Text. That’s not all it can do though, as you are about to find out in this tutorial!
Note: If your GUI application is in need of Multi-Line Text input, the PyQt6 QTextEdit widget is what you are looking for.
You can find a complete list of methods, signals and options available for the QLineEdit at the end of this tutorial.
Creating a QLineEdit Widget
Below is the “base” implementation of a simple QLineEdit Widget.
We will create the QLineEdit widget by importing the QLineEdit Class from the QtWidgets module in PyQt6. It needs no parameters, except for the parent in which it’s located. (In this case, our Window is the parent, hence we just pass in “self”).
from PyQt6.QtWidgets import (
QApplication, QWidget, QLineEdit
)
from PyQt6.QtGui import QIcon
from PyQt6.QtCore import Qt
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.resize(300, 250)
self.setWindowTitle("CodersLegacy")
self.input = QLineEdit(self)
self.input.move(80, 100)
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
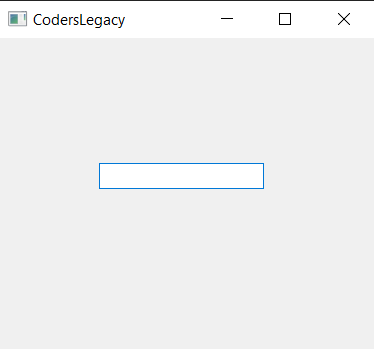
Retrieving QLineEdit values
In order to retrieve the entered text from the QLineEdit
widget, you have to use the text()
method on it. There are many different ways to use this method, either with the use of QLineEdit
‘s inbuilt functions or by using another widget.
As a supporting widget in this example, we will use the QPushButton widget. We’ve created a submit button that calls the function show
that uses the text()
method on our QLineEdit
object.
from PyQt6.QtWidgets import (
QApplication, QWidget, QLineEdit, QPushButton, QVBoxLayout
)
from PyQt6.QtCore import Qt
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.resize(250, 250)
self.setWindowTitle("CodersLegacy")
layout = QVBoxLayout()
self.setLayout(layout)
self.input = QLineEdit()
self.input.setFixedWidth(150)
layout.addWidget(self.input, alignment= Qt.AlignmentFlag.AlignCenter)
button = QPushButton("Get Text")
button.clicked.connect(self.get)
layout.addWidget(button)
button = QPushButton("Clear Text")
button.clicked.connect(self.input.clear)
layout.addWidget(button)
def get(self):
text = self.input.text()
print(text)
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
We’ve also thrown in a bonus Clear
button that calls the clear()
method on the QLineEdit
widget. This deletes all the contents of the QLineEdit
widget.
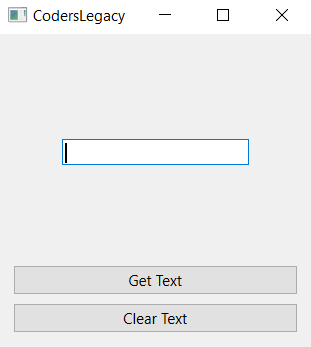
Try running this code for the PyQt6 QLineEdit Widget yourself to understand it better.
Other QLineEdit Methods
Here are few additional code examples that cover the most important and useful QLineEdit features.
Taking passwords from User
The setEchoMode()
takes several different ” echo modes”, one of which is the password mode. This obscures the password and adds an extra layer of security.
There is only really one additional command we have to call, shown in the code below. Just add this line to any of our previous examples, and you will see the magic!
self.input.setEchoMode(QLineEdit.EchoMode.Password)
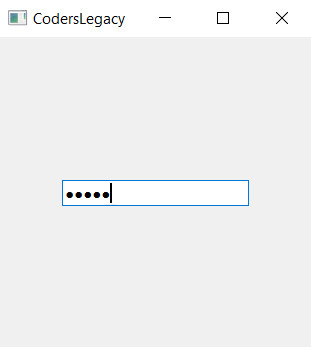
Using QLineEdit Signals
We don’t have to rely on a Button to trigger functions to use on the QLineEdit widget. PyQt6 introduces a system of signals and slots, which allow us to connect functions to certain events, such as when the user types in a character. Such a mechanic can be used in a variety of ways, such as validation.
There are several signals available for the QLineWidget that you can find in a table at the end of the tutorial.
Their usage is very straightforward. The format is:
widget.signal_name.connect(function_name)
In the below example, we will have an error message popup if the User attempts to type in something that is not an alphabet. We are using the signal called “textChanged”, which triggers whenever the text is modified in any way (new character added, a character removed, etc.)
from PyQt6.QtWidgets import (
QApplication, QWidget, QLineEdit, QPushButton, QVBoxLayout
)
from PyQt6.QtCore import Qt
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.resize(250, 250)
self.setWindowTitle("CodersLegacy")
layout = QVBoxLayout()
self.setLayout(layout)
self.input = QLineEdit()
self.input.setFixedWidth(150)
self.input.textChanged.connect(self.check)
layout.addWidget(self.input, alignment= Qt.AlignmentFlag.AlignCenter)
def check(self):
for x in self.input.text():
if x < 'a' or x > 'z':
print("Invalid Character entered")
return
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
Run the code yourself to see the output!
QLineEdit Methods
A complete list of each method for QLineEdit widgets with a brief description.
Methods | Description |
---|---|
setAlignment() | Decides the Alignment of the text. Takes 4 possible values, Qt.AlignLeft, Qt.AlignRight, Qt.AlignCenter and Qt.AlignJustify. |
clear() | Deletes the contents within the QLineEdit widget. |
setEchoMode() | Controls the mode for text appearance in the widget. Values are QLineEdit.Normal, QLineEdit.NoEcho, QLineEdit.Password, QLineEdit.PasswordEchoOnEdit. |
setMaxLength() | Defines the maximum number of characters that can be typed into the widget. |
setReadOnly() | Makes the widget non-editable |
setText() | The text passed to this method will appear in the widget. |
text() | Returns the text currently in the widget. |
setValidator() | Defines the validation rules. |
setInputMask() | Applies mask of combination of characters for input |
setFont() | Sets the font for the widget. |
setFixedWidth(width) | Sets the maximum width for the Widget in pixels. |
QLineEdit Signals
A list of signals available for the QLineEdit widget.
Signals | Description |
---|---|
textChanged() | A signal that activates when the text in the QLineEdit widget is changed in any manner. |
textEdited() | Almost the same as textChanged() , however this signal is not emitted when the text is changed programmatically, for example, by calling setText(). |
editingFinished() | This signal is emitted when the Return or Enter key is pressed or the line edit loses focus (when it’s no longer highlighted) |
inputRejected() | Emits a signal when the user inputs a character that’s considered “invalid”. This signal is used together with the Validator functions. |
returnPressed() | Emitted when either the return key or enter key is pressed by the user. |
Interested in learning more about PyQt6 and it’s various widgets? Follow the link!
This marks the end of the PyQt6 QLineEdit Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.