A compilation of all good coding practices for both new and experienced programmers. A must read, especially for newcomers or those looking to begin develop proper software and programs, even if it’s only on a basic level.
In some sections of this article we have some code snippets for further elaboration on certain coding practices. In order to make sure this article remains accessible to programmers of all languages, we have utilized a form of pseudocode so that it may be understood. It should do well enough to get the general idea across to you.
Picking the right language
The first and most important step before beginning a project is picking the right language for it. The last thing you want is to realize halfway through that your language of choice is unable to meet the requirements of the project. Do some research, ask the right people and map out all the requirements of your project.
What should you look for while picking a programming language? Other than the fact that it’s even suitable for your project, you should also consider the platforms your targeting. Lets discuss mobile devices. Not all programming languages are very suited to be run on phones so if you’re targeting the mobile sector, you’ll need a language that works well in that environment.
There are other factors to consider, like the community. The larger the community, the easier it is to get help, and the more support that language will have. There’s also the learning curve to consider. If you’re a new programmer, it’s best to stick to something simpler even if it comes at a slight cost. Experienced programmers, due to transferable skills, shouldn’t face the same issue.
The above advice does not always apply in certain scenarios. For instance, simple programs and scripts. Large projects however, are another story.
Naming Variables
Variable naming is one of the coding practices that one must always abide by in a proper project or team. Known commonly as the naming convention, this has a great effect on the readability of your code. Lets look through some examples of variables names in the pseudocode below.
The input() is meant to represent us taking input from the user
x = input()
y = input()
z = x * y
print(z)
len = input()
width = input()
area = len * width
print(area)
Compare the two, and decide for yourself which one is easier to read. In a large program, with hundreds of variables, you can easily get confused if you haven’t given your variables meaningful names. Remember, one day you will be looking back on your code, and it might not make sense.
Some tips on naming variables.
- Keep your variable names short. Use abbreviations where you can, such as len for length.
- Stay consistent. For instance, use only lowercase characters, instead of a mix of lower and uppercase. Most languages are case sensitive, so you if you forget the right combination of lower and uppercase, you’ll see an error. Keep things simple.
- if your using multiple word variables name, separate them using the
_
character.
Comments
Comments are just pieces of text that are ignored by the compiler during execution. So as far as the program is concerned, the comment may as well not exist. Comments use special characters which declare them as comments. This character varies from language to language, but we’ll list down some in the examples below.
#This is a comment in Python
//This is a comment in PHP and Java
'This is a comment in VB.NET
Making your code readable and using meaningful variable names will only take you so far. Sometimes the name will not be enough to describe the function of a variable, or maybe you used a complex function or calculation that’s not very easy to understand. Or maybe you want to leave a few notes for your future self or even the user.
This is where comments come in. Comment aren’t random pieces of text, they are meant to explanatory. Comments are inserted strategically amongst the code to help the reader understand the purpose of the code without too effort. It’s important to achieve a balance between the number of comments you insert. Don’t insert them into places which do not require explanation neither should you be scarce while adding them.
While adding comments, it’s best to do so while you’re writing the code itself. It’s not uncommon for the programmer himself to forget the purpose of a specific line, much less some other programmer or user. In fact, that is the purpose of a comment, to capture your exact thoughts while you were writing that line(s).
Comments are one of the most important coding practices, and often separate a good programmer from an amateur.
Documentation
First of all, what is documentation? Any written text, diagrams, images or videos that describe a software or program is called documentation. There are several different types of documentation. One type of documentation is meant for the end user. This type of of documentation focuses on explaining how to use the front end of the software or program. We see this commonly in the form of user manuals that come along the software we download.
The other type of documentation is focused towards the programmer. This describes the back end of the program, it’s dependencies, the logic used behind it, etc. This kind of documentation especially comes in handy when a new programmer is hired to manage the software. As he wasn’t present during the initial construction of the software, he needs to be informed of the inner workings. This is where documentation comes in. You will see this commonly in open source software, where there are dozens of programmers working side by side, or in large software houses who spend most their time managing and improving software that they have already built. (Google and Microsoft).
What does this mean for you? When making software (actual software, not a 10 line script) documentation is almost compulsory. There are hundreds of little things involved in constructing the program logic, and chances are you won’t be able to remember all of them within a few weeks. Even worse, you leave the software incomplete for a while, come back a month or two later, and you’ve forgotten everything about it. You’ll wonder, “what does this variable do?”, “Why is this line here?” etc. You’ll likely spend days, if not weeks simply re-figuring out your old code. However, if you have documentation present, you could begin coding again in the space of a few hours.
Documentation, as mentioned above, can be anything that explains the program code. So there is no set method to creating documentation. Everyone has their own style. Creating good and helpful documentation is a skill that will come with practice.
Indentation:
The importance of indentation can vary from language to language. In some, it’s an integral part of the syntax, where as in others indentation has no effect on the syntax. One thing however remains common throughout all languages is that indentation significantly improves the readability of your code.
Be mindful of how much you indent. Some programmers prefer to use spaces to indent, some prefer to use Tab spaces. Whichever you pick, stay consistent in that style.
Keeping the code simple
Another important aspect here is pre planning. In large scale projects, it’s not uncommon to spend days of simply planning how to approach the project, diving big problems into smaller problems more managable problems or creating flowcharts and diagrams (depending on the type of software) etc. Even if you’re doing something on a small scale, a few hours of prep before the actual coding will help alot. All this helps to make sure you maintain a clear goal while coding, preventing your coding from getting all jumbled up and confusing.
Even in the midst of coding, you should take a break every now and then and think about what you’re really doing. It’s a common saying amongst programmers that writing code is only 20% of a programmer’s job. The rest is spent in planning, debugging and documentation.
Avoid using fancy solutions unless you really know what you’re doing. Simplicity is key to coding. Not only is it easy to read, but easy to build upon as well. Code maintenance is a very real thing. The easier the code is to understand, the easier maintenance will be.
Portability
If you’re making a cross platform software, then this is pretty obvious. But even on the same platform, such as PC’s, portability is a very real issue. There are three main OS’s used in PC’s (Windows, Linux and Mac) and each have their own quirks. Some Operating systems like Linux also require special dependencies, some libraries may not work on certain operating systems. You have to do some in-depth research as to the third party resources you’re using. As long you as use the standard library, there shouldn’t be any issues though.
Portability is also a issue when it comes to PC’s of the same OS. Let’s look at the some psuedocode below.
path = "C://Users/CodersLegacy/MySoftware/savefile.txt"
file = open(path, 'write')
The code above shows that the programmer’s intention was to create a save file for his software at the above destination. However, what he failed to account is that only his PC will have the above File path. This is what we call a fixed file path. And unless the exact same file path is found in the user’s PC, the program will not work. This won’t happen as the User name is bound to be different, and the software could be installed in a different drive. Instead, you have to use functions to find the location of the software, and then proceed to save the file there. Something like the pseudocode below.
path = getfilepath() + "savefile.txt"
file = open(path, 'write')
The getfilepath
is just a dummy function we based of the getcwd()
function from python which returns the directory of the .exe
or .py
file. Hence, the above codes places the save file in the required folder, regardless of the PC or arrangement of file paths.
Of course, portability issues extends to many other things besides just file paths. It’s good practice to test your code on several devices during development to ensure portability. Sometimes unique issues that may not be present on your PC will come forward. There are too many factors to list down here.
A little note on portability between different OS’s. Some OS’s may not natively support certain libraries or third party tools. So before including any such thing, make sure to check whether it works across multiple OS’s (if cross platform software is what you’e aiming for).
Exception Handing
Another easy way to differentiate between an amateur and an experienced programmer is how their implementation of exception handling in their code. So what are exceptions and what makes them one of the most important coding practices?
An exception refers to an event that is triggered during the execution of a program that goes against the flow, disrupting the program. An exception in Python is meant to represent a error that occurs due to Python being unable to handle an unexpected situation. And due to python being unable to handle this situation, it terminates the program.
So what’s the issue here? Besides the fact that an error is being thrown. In a large scale software, such as a Video game, you can expect hundreds errors to be thrown over it’s lifetime. How would your customers feel if their game was shutdown after each error? This where exception handling comes in. Instead of letting the error crash your program, you “handle” it instead, allowing for a different outcome to be reached. The exact syntax varies from language to language but the general idea remains the same. Below is of exception handling from Python.
x = 5
try:
x = x / 0
except:
x = 0
print("ZeroDivisionError detected, x set to 0")
The following code will not crash when the ZeroDivisionError occurs, instead it will simply divert itself to the code block under the except
statement and continue onwards.
Logging
Like exception handling, a good logging implementation is another sign of a good programmer. Often in the midst programming, the programming will have the values of variable displayed to screen using a print function or equivalent. This is done to monitor how the variable is changing during the execution of the program, a form of debugging. However, this is not the correct way of doing it, despite common belief.
While doing so at a basic level is fine, most programmers fall into the habit of using print functions and carry on this habit into more advanced scenarios, such as software development. The correct method is the use of logging.
Logging is act of saving system events in a file. These “logs” track your program activities and events that occur during execution. All these are saved to a single file, which we call, a “log” file. This file can then be used for troubleshooting or debugging .
One of the many benefits of the logging system is that you don’t have to go around deleting all the print statements that you wrote. A logging system may be included in the final product for additional debugging purposes. Furthermore, you get a saved copy of the all system events (if implemented properly) that you can view later, unlike the print statement, which only lasts for a single execution. The logging functions can also log more than just text and numbers. They can log system events at the exact time that they occur. This is particularly useful while debugging.
Logging varies from language to language, so only it’s general concept as one of the many coding practices was discussed here. You can look it up in your respective language later.
DRY Principle
The DRY principle, or full form, the Don’t Repeat Yourself principle is another one of those good coding practices that are a must know. The DRY principle is actually directed towards the use of functions in programmers. If you remember, functions are re-usable blocks of code. Without them we would have to repeat the same block of code many times, increasing redundancy.
Many people might say that anything that repeats should be converted into a function. However, it’s better to maintain a balance. The limit is 3. If anything is repeating more than 3 times, make a function. There is no need to create a function for anything that’s repeating twice or looks similar.
Keep in mind, a function is not always a solution. Sometimes you need to simply review your code and come up with a smarter solution.
File Structure
File structure comes is something comes into play when you graduate from creating single file programs. Maintaining a good and understandable file structure during software development is important. We’ll be using the below image to explain what we mean by good File Structure.
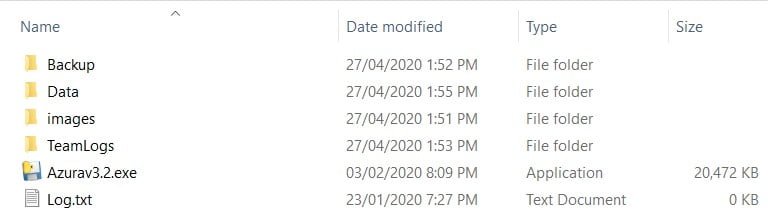
In the above example, you can an exe file, with a text file meant for logging purposes next to it. It doesn’t matter much where you keep the log. I’ve seen it both ways. Moving on, you can see individual folders for images and Data. The data folder contains some text files which contain data which the program requires. The TeamLogs is folder meant to hold many logs for individual components in the software.
Whats the take away here? Create separate folders like we did above to keep things organized. Separate folders for images, videos, dependencies, scripts etc. Create a file structure that makes sense for your program.
Conclusion
Before beginning a project, plan out your code properly. Decide your language, any dependencies you’ll need, the platform you’ll be targeting etc. Decide a naming convention you’ll use for naming variables.
While coding, make sure to include an exception handling and logging system. Follow the DRY principle and make sure to write your code in such a manner that it remains portable. Make sure to research properly any additional libraries or tools you are using.
Finally, in your ending stages, don’t forget to leave comments in appropriate areas and finally create some documentation for your code. The size and complexity of the documentation will depend on the type of software. Not all software will require a user manual for one.
Always remember these practices before you begin coding, even if it’s just for a small project. It’s best to get into a habit of following these early on.
This marks the end of our Coding Practices article. If there were any other great coding practices we may have missed out on, feel free to share! Any relevant questions can be asked in the comment section below.