This a tutorial on the JavaFX DatePicker component.
The JavaFX DatePicker is a GUI component that, through the use of a popup calendar allows the user to select a Date. The popup looks exactly like a regular calendar with valid dates and proper sorting. You can alternatively type in the Date of your choice in the entry field.
Creating a DatePicker
To create a DatePicker object, you just have to call the DatePicker class without any input parameters. You can add this object into the layout to display it.
You can also create a DatePicker object by passing a LocalDate object into the DatePicker class parameters.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.DatePicker;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
DatePicker datepick = new DatePicker();
VBox layout = new VBox(datepick);
Scene scene = new Scene(layout, 200, 250);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
The GUI output of the above code:
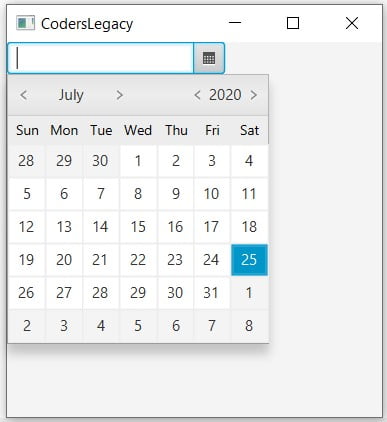
Get Dates from DatePicker
We’re going to create a label onto which the date we select will be displayed. Using the event handling, setOnAction()
function we cause the selected date to be returned using getValue()
. This function will be called every time the user selects a date.
Finally, using setText()
we set the returned date value to the Label. It’s necessary to have a string portion present in the setText()
, else an error will be thrown.
Label label = new Label(" ");
DatePicker datepick = new DatePicker();
datepick.setOnAction(e -> {
label.setText("Date: " + datepick.getValue());
});
VBox layout = new VBox(datepick, label);
layout.setSpacing(240);
The output of the above code is shown below. Keep in mind that the getValue() function returns a value of datatype LocalDate
.
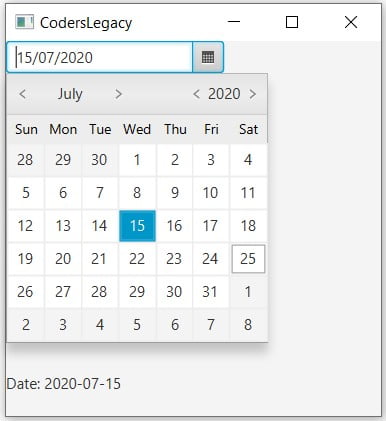
DatePicker methods
A list of important and useful methods for the DatePicker widget.
Method | Description |
---|---|
setValue() | Sets a value for the DatePicker. Must be of appropriate type. |
getValue() | Returns the current selected value. |
setShowWeekNumbers() | Takes a True or False value, based of which it shows the week numbers next to the date. Default value: False. |
isShowWeekNumbers() | Returns True or False depending on the whether the week numbers are showing. |
You can learn more about the DatePicker Class and it’s method from the official documentation.
Other JavaFX Widgets:
This marks the end of the JavaFX DatePicker Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.