This article is a list of widgets available in PyQt5.
Each widget in PyQt5 is included here with a brief description regarding it’s use and purpose. This is followed by a code sample from the widget’s respective article and the output. The usage is shown through the use of an image or video.
If you’re an absolute beginner to PyQt5, you should read this beginner guide before trying to learn any of the widgets below.
Python PyQt5 Widgets:
QLabel
QLabel is one of the simplest widgets in PyQt5 with the ability to display lines of text. This widget comes with many supporting functions and methods to allow us to retrieve,update and format this text whenever we want.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,500,300)
win.setWindowTitle("CodersLegacy")
label = QLabel(win)
label.setText("GUI application with PyQt5")
label.adjustSize()
label.move(100,100)
win.show()
sys.exit(app.exec_())
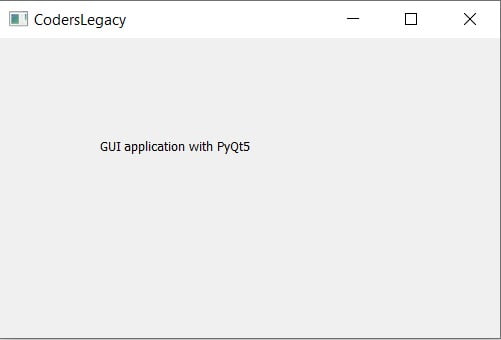
QPushButton
One of the most basic and common widgets in PyQt5, is QPushButton. As the name implies, it’s a button that triggers a function when pushed (clicked).
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,500,300)
win.setWindowTitle("CodersLegacy")
button = QtWidgets.QPushButton(win)
button.clicked.connect(win.close)
button.setText("Quit Button")
button.resize(120,60)
button.move(350,220)
win.show()
sys.exit(app.exec_())
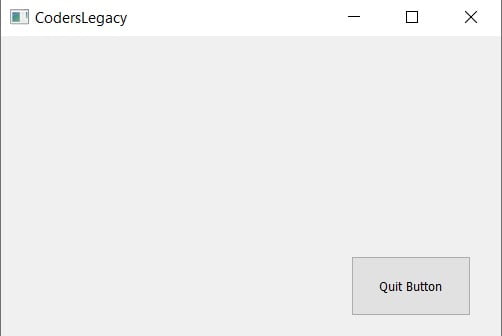
QLineEdit
Every GUI needs a way of taking input from the User. In PyQt, the most common way of taking input is through the QLineEdit widget. It offers you a single line where you can input Text.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
def show():
print(line.text())
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
line = QtWidgets.QLineEdit(win)
line.move(100,80)
win.show()
sys.exit(app.exec_())
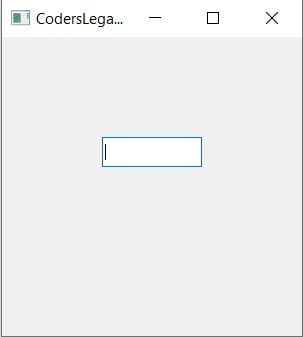
QRadioButton
Radio Buttons are commonly seen in GUI’s where the user is presented with a list of options. Unlike a CheckBox, only one Radio Button out of many (group) can be picked.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow, QGridLayout
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
layout = QGridLayout()
radio = QtWidgets.QRadioButton(win)
radio.setText("Option1")
radio.move(50,100)
layout.addWidget(radio, 0, 0)
radio2 = QtWidgets.QRadioButton(win)
radio2.setText("Option2")
radio2.move(50,150)
layout.addWidget(radio, 0, 1)
win.show()
sys.exit(app.exec_())
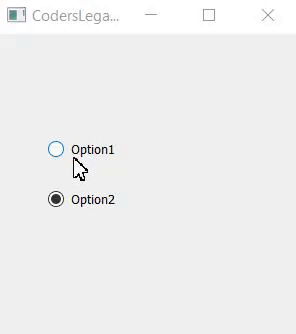
QCheckBox
A Checkbox is an important part of any GUI, used when you want to present the User with a list of options. In PyQt5, we have a widget called the QCheckBox which we use to create check boxes or check buttons as they are sometimes called.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow, QGridLayout
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
layout = QGridLayout()
check = QtWidgets.QCheckBox(win)
check.setText("Option1")
check.move(50,100)
layout.addWidget(check, 0, 0)
check2 = QtWidgets.QCheckBox(win)
check2.setText("Option2")
check2.move(50,150)
layout.addWidget(check2, 0, 1)
win.show()
sys.exit(app.exec_())
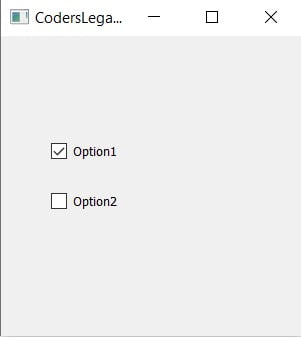
QComboBox
A QComboBox widget presents a drop-down list of items for the user to select an option from. The advantage of this widget is that it takes up very little space on the screen, even if it has alot of different options. This is the widget to be used in space restricted GUI programs.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
combo = QtWidgets.QComboBox(win)
combo.addItem("Python")
combo.addItem("Java")
combo.addItem("C++")
combo.move(100,100)
win.show()
sys.exit(app.exec_())
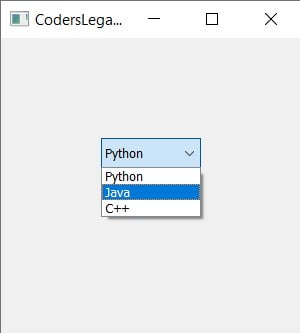
QTextEdit
Like the QLineEdit widget, QTextEdit is used to take input from the user in the form of text. However, unlike QLineEdit which only takes input in a single line, QTextEdit offers a large area where the User can input several lines of text, or even several paragraphs.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QWidget
import sys
app = QApplication(sys.argv)
window = QWidget()
window.setGeometry(400,400,300,300)
window.setWindowTitle("CodersLegacy")
date = QtWidgets.QTextEdit(window)
window.show()
sys.exit(app.exec_())
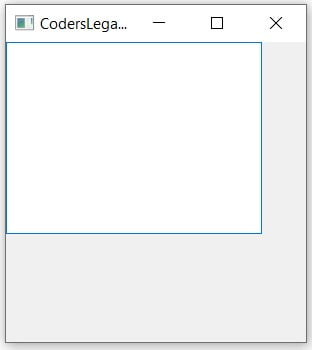
QMessageBox
QMessageBox is a useful PyQt5 widget used to create dialogs. These dialogs can be used for a variety of purposes and customized in many ways to display different messages and buttons.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow, QMessageBox
import sys
def show_popup():
msg = QMessageBox(win)
msg.setWindowTitle("Message Box")
msg.setText("This is some random text")
msg.setIcon(QMessageBox.Question)
msg.setStandardButtons(QMessageBox.Cancel|QMessageBox.Ok)
msg.setDefaultButton(QMessageBox.Ok)
msg.setDetailedText("Extra details.....")
msg.setInformativeText("This is some extra informative text")
x = msg.exec_()
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
button = QtWidgets.QPushButton(win)
button.setText("A Button")
button.clicked.connect(show_popup)
button.move(100,100)
win.show()
sys.exit(app.exec_())
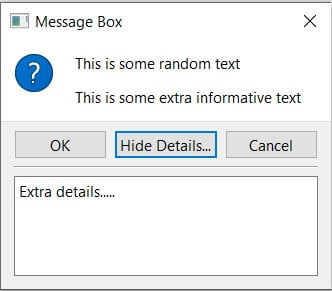
QSlider
The PyQt QSlider widget provides the user with a GUI friendly way of picking a value from a range of different values. The Slider widget has a handle that you can move across a groove. As you move the slider, the selected value changes accordingly.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
from PyQt5.QtCore import Qt
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
slider = QtWidgets.QSlider(Qt.Horizontal, win)
slider.setGeometry(50,50, 200, 50)
slider.setMinimum(0)
slider.setMaximum(20)
slider.setTickPosition(QtWidgets.QSlider.TicksBelow)
slider.setTickInterval(2)
win.show()
sys.exit(app.exec_())
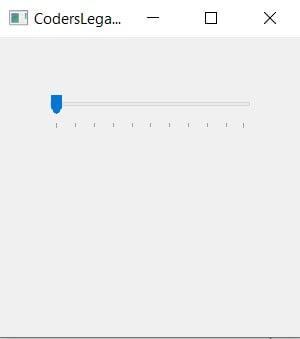
QInputDialog
Alot of GUI’s come with prompts in one or the other. One common use of such prompts or “dialogs” are for taking input from the User. PyQt5 has it’s QInputDialog widget which allows us to create a variety of different input dialogs to take input in many different ways.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QWidget, QInputDialog, QLineEdit, QPushButton, QLabel
import sys
def display():
text , pressed = QInputDialog.getText(window, "Input Text", "Text: ",
QLineEdit.Normal, "")
if pressed:
label.setText(text)
label.adjustSize()
app = QApplication(sys.argv)
window = QWidget()
window.setGeometry(400,400,250,250)
window.setWindowTitle("CodersLegacy")
label = QLabel(window)
label.setText("Empty Text")
label.move(60,80)
button = QPushButton(window)
button.setText("Press me")
button.clicked.connect(display)
button.move(60,120)
window.show()
sys.exit(app.exec_())
The image below is just one of the many possible dialogs that can be created for Input.
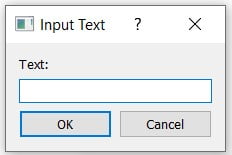
QProgressBar
Progress Bars are easily one of the most interesting PyQt5 widgets mentioned in this list. They are a great way to visualize progression of computer operations such as file transferring, downloading, uploading, copying etc. The alternative would be using text which is rather boring in comparison.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
prog_bar = QtWidgets.QProgressBar(win)
prog_bar.setGeometry(50, 50, 250, 30)
prog_bar.setValue(0)
win.show()
sys.exit(app.exec_())
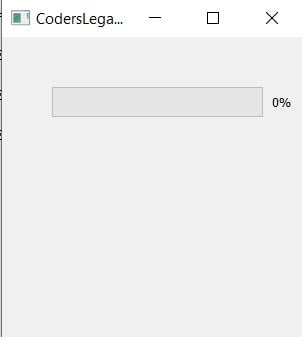
QPixmap
PyQt5, with the QPixmap widget brings in support for displaying images in your GUI window. An image is first loaded in QPixmap. The label widget is actually responsible for displaying this image, which is done by passing the pixmap object to the label.
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel
from PyQt5.QtGui import QIcon, QPixmap
from PyQt5 import QtCore
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,500,400)
win.setWindowTitle("PyQt5 Widgets")
label = QLabel(win)
pixmap = QPixmap('Kitties.jpg')
label.setPixmap(pixmap)
label.adjustSize()
win.show()
sys.exit(app.exec_())
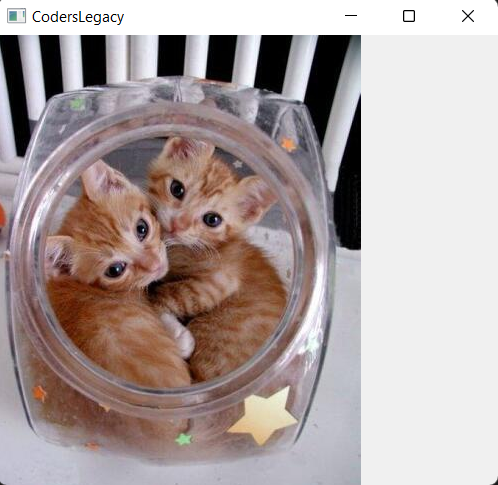
QTableView
PyQt6 provides us with the QTableWidget which has the functionality required to create spreadsheets and tables. Easily one of the best PyQt Widgets in this List!
from PyQt5.QtWidgets import QApplication, QWidget,
QTableWidget, QTableWidgetItem,
QVBoxLayout
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQt5 QTableView")
self.setGeometry(500, 400, 500, 300)
self.Tables()
self.show()
def Tables(self):
self.tableWidget = QTableWidget()
self.tableWidget.setRowCount(5)
self.tableWidget.setColumnCount(3)
self.vBox = QVBoxLayout()
self.vBox.addWidget(self.tableWidget)
self.setLayout(self.vBox)
App = QApplication(sys.argv)
window = Window()
sys.exit(App.exec())
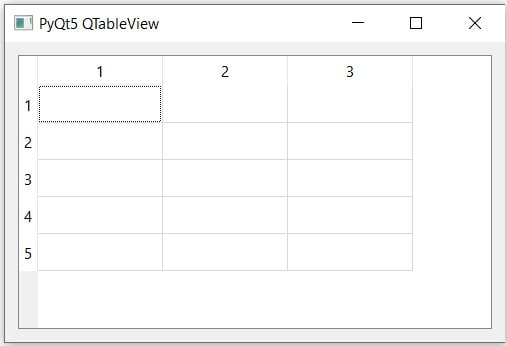
Do you have any additional suggestions for our PyQt Widgets List? Let us know in the comments section below.
This marks the end of the PyQt5 List of widgets article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.