In this tutorial we will discuss the wxPython GridBagSizer Layout Sizer.
The wxPython GridBagSizer is one of the five sizers in wxPython designed to help with the layout management of widgets in the window. The GridBagSizer is a variant of the FlexGridSizer, from which it inherits. It has the added functionality of allowing widgets to be placed in specific “cells”.
Instead of just adding widgets sequentially, the GridBagSizer makes you place them with a specific row and column number. This allows you to even leave some “cells” blank.
wxPython GridBagSizer Example
In this example we’ll create a simple Keypad using the wxPython GridBagSizer.
wrapper = wx.BoxSizer(wx.VERTICAL)
First we created a BoxSizer to act as a wrapper around the GridBagSizer. The idea is to use the BoxSizer to create some padding between the widgets in the GridBagSizer and the edges of the window. (Try it both ways, with and without the wrapper).
sizer = wx.GridBagSizer(5, 5)
Next we create the GridBagSizer, passing in 5 and 5 in the parameters for 5 rows and columns each.
sizer.Add(wx.StaticText(panel, label = "A Keypad"), flag = wx.ALIGN_CENTRE, pos = (0, 1))
Here we add in a StaticText widget into the Sizer. The first parameter is the widget, followed by a flag. This flag is used to center-align the widget. The third parameter is the pos
. We want to place this text in the center-top of the window, so we place it in the first row and the middle column.
sizer.Add(wx.Button(panel, label = "1"), flag = wx.EXPAND, pos = (1, 0))
sizer.Add(wx.Button(panel, label = "2"), flag = wx.EXPAND, pos = (1, 1))
sizer.Add(wx.Button(panel, label = "3"), flag = wx.EXPAND, pos = (1, 2))
...
...
We then proceed to add in 9 buttons to serve as keys. We also make use of the wx.EXPAND
flag so that they expand to fill all available space.
wrapper.Add(sizer, 1, wx.EXPAND | wx.ALL, 10)
panel.SetSizer(wrapper)
Lastly we add the GridBagSizer into the BoxSizer, with the appropraite flags. A border of 10 to serve as padding, and the wx.EXPAND
flag so that the Sizer can grow. (Widgets won’t grow if the Sizer is unable to grow).
The complete code:
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
panel = wx.Panel(self)
wrapper = wx.BoxSizer(wx.VERTICAL)
sizer = wx.GridBagSizer(5, 5)
sizer.Add(wx.StaticText(panel, label = "A Keypad"), flag = wx.ALIGN_CENTRE, pos = (0, 1))
sizer.Add(wx.Button(panel, label = "1"), flag = wx.EXPAND, pos = (1, 0))
sizer.Add(wx.Button(panel, label = "2"), flag = wx.EXPAND, pos = (1, 1))
sizer.Add(wx.Button(panel, label = "3"), flag = wx.EXPAND, pos = (1, 2))
sizer.Add(wx.Button(panel, label = "4"), flag = wx.EXPAND, pos = (2, 0))
sizer.Add(wx.Button(panel, label = "5"), flag = wx.EXPAND, pos = (2, 1))
sizer.Add(wx.Button(panel, label = "6"), flag = wx.EXPAND, pos = (2, 2))
sizer.Add(wx.Button(panel, label = "7"), flag = wx.EXPAND, pos = (3, 0))
sizer.Add(wx.Button(panel, label = "8"), flag = wx.EXPAND, pos = (3, 1))
sizer.Add(wx.Button(panel, label = "9"), flag = wx.EXPAND, pos = (3, 2))
sizer.AddGrowableRow(1)
sizer.AddGrowableRow(2)
sizer.AddGrowableRow(3)
sizer.AddGrowableCol(0)
sizer.AddGrowableCol(1)
sizer.AddGrowableCol(2)
wrapper.Add(sizer, 1, wx.EXPAND | wx.ALL, 10)
panel.SetSizer(wrapper)
self.Centre()
self.Show()
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
The output of the above code:
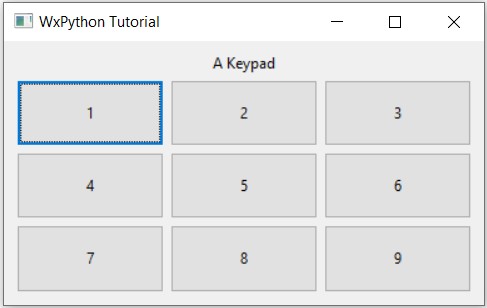
Other Sizers in wxPython
There are many other Sizers in wxPython that you can use instead of GridBagSizer. In fact, the best layouts are often the result of combining together several different types of Sizers together. This can be done by simply nesting them into each other. There is no limitation to this ability.
This marks the end of the wxPython GridBagSizer Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.