This article compares two Python GUI libraries, PyQt vs Tkinter.
The PyQt vs Tkinter debate is one I’ve been seeing ever since I joined up with several Python communities and social media sites. In this article, I’m going to share my own journey and personal experience with these two GUI libraries.
Besides my personal experience, I’ve included a side by side comparison of Tkinter and PyQt GUI’s with the same widgets. Most GUI’s have the same widgets like Labels, Buttons, CheckButtons and Menu’s so this makes the comparison a lot easier.
By the end of this article, the difference between these two libraries will be made clear. If you haven’t begun using either yet, this article will help you make that decision.
Tkinter
First Impressions
Tkinter was the first GUI library I started out with. I was unaware of any other GUI libraries like PyQt. I used Tkinter for many simple projects, and the experience was pretty good. It’s part of the Python Standard Library so you don’t have to worry about downloading and installing it either. It was simple to learn, and by the end of it I could create a simple GUI with several functioning widgets in under 10 mins.
Widgets
Even before I came across other GUI libraries, Tkinter’s widgets felt rather old fashioned. They remind me of the kind of GUI I would see in software of the 2000’s. Another issue I had with Tkinter was a slight screen blurriness issue for which I had to use the ctypes library to fix.
Putting aside the looks however, Tkinter has a fairly large number of widgets and support for menus. One widget that’s rather unique to Tkinter is the Canvas widget which is like a drawing board where you can display images and draw graphs etc. Overall, Tkinter does fairly well in the widgets category if you put the looks aside.
Miscellaneous
Tkinter
has a special module called ttk
which was released alongside Tkinter 8.5
. The ttk
module has it’s own Button, Check Button, Entry, Frame and more Widgets. Importing this module will automatically overwrite the Tkinter variants. The ttk
widgets have a more modern and sleek look to them and are fully compatible with Tkinter.
You can begin learning Tkinter with our Tkinter Tutorial Series.
PyQt
First Impressions
As my programming knowledge and outreach grew, I began to hear about other GUI libraries. I would sometimes come across posts and questions by people asking for a library with a more “modern” look than Tkinter. Other experienced dev’s would often recommend PyQt as the better alternative, leading to me begin my PyQt journey.
The first thing I noticed with PyQt that the number of tutorials and guides for it were considerably lower than that for it’s competitor Tkinter. In fact, a good number of these tutorials were actually for the outdated PyQt4, instead of it’s newer PyQt5 version. This is actually a factor that drove me to develop my own Tutorial series on PyQt.
Another experience I has while coding is that PyQt5 is harder to debug than Tkinter. Sometimes if there was a syntax error or bug present in my code, the GUI window would just shutdown with no warning and no error message. This did however became less of an issue as I became more familiar with the library and it’s syntax.
Furthermore the initial startup time for a PyQt5 application is actually more than a Tkinter application of similar size. Despite only a few widgets present, PyQt5 would sometimes take almost twice the time to display compared to it’s Tkinter equivalent.
Widgets
Now, onto the actual PyQt code. My first impression of it was that it was significantly lengthy as compared to Tkinter. Creating and customizing a single widget in PyQt could take up more than 5 lines. Whereas in Tkinter, I would require 3 lines max. In PyQt’s defense however, it’s lines were shorter and simpler to understand (individually) so it sort of balances out.
The difference in GUI’s was pretty clear. Even before I created any widgets, looking at the PyQt display window, I could tell that it was superior. It looked cleaner and sharper unlike Tkinter which gave me this blurry kind of look. If I had to describe PyQt’s window in a single word, it would be “sleek”.
PyQt5 also has a larger number of number of widgets. Most of widgets of PyQt and Tkinter are the same, but PyQt wins out in the “special widgets” category. Examples of these are the QProgressBar, QSpinBox, QDial, QDateEdit etc.
Another strong advantage of PyQt5 is it’s CSS support. You can use CSS on PyQt5 components to style them and change many things about their appearance.
Miscellaneous
PyQt has a special (and popular) tool called Qt designer which provides you with an GUI editor that you can use to create your own GUI’s using Qt Widgets. It’s like a drag and drop editor where you don’t really have to code, rather just manipulate the widgets and their placement in the window.
Widget Comparison
Below are comparisons between three widgets that both GUI libraries have in common. I picked the three that showed the greatest difference.
Comparison between the QLineEdit and Entrybox.
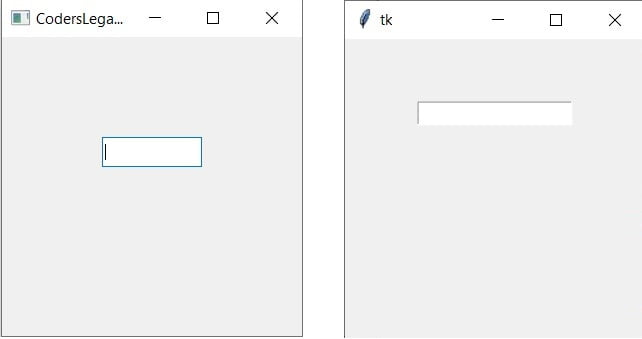
QPushButton and Button Comparison (Biggest difference is here in my opinion).
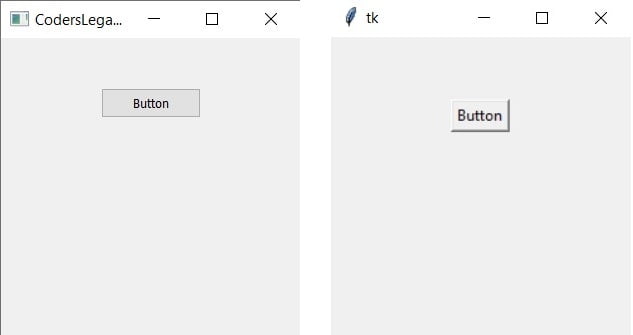
Comparison between QComboBox and ComboBox.
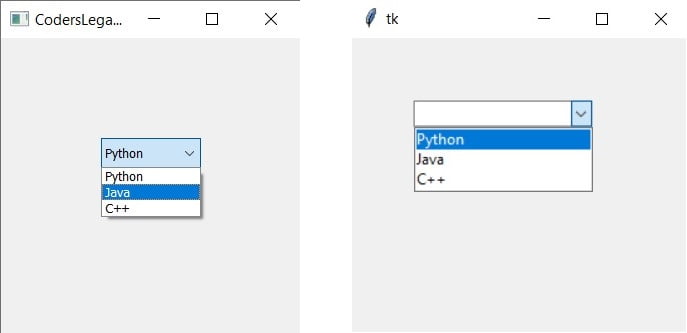
In can be a bit hard to tell the difference by simply comparing widgets individually like this. Ideally you should try out both. You’ll understand the difference real quick then.
PyQt’s widgets are more interactive, such as the button glowing blue when the cursor is over it. You can only tell such a thing when actually using the GUI for yourself, so it’s best to actually run code for yourself. It’s these little differences that combined, end up making a big difference.
PyQt vs Tkinter – Code Comparison
Just for the sake of it, we decided to throw in a code comparison as well. The code below will create the same GUI with the same widgets. It’s purpose is simple. There is an introductory label, a entry field which takes input and there are two buttons, one which prints the user input and the other closes the application.
It’s a fairly simple GUI application that any beginner might make. The code should be easy enough for you to follow even if you don’t know anything about either library.
Tkinter
from tkinter import *
def display():
print(my_entry.get())
def quit_window():
root.destroy()
sys.exit()
root = Tk()
root.geometry('300x300')
my_label = Label(root, text = "Tkinter GUI Application")
my_label.pack(pady = 10)
my_entry = Entry(root)
my_entry.pack(pady = 20)
my_button = Button(root, text = "Print", command = display, width = 10)
my_button.pack(pady = 10)
my_button2 = Button(root, text = "Quit", command = quit_window, width = 10)
my_button2.pack(pady = 10)
root.mainloop()
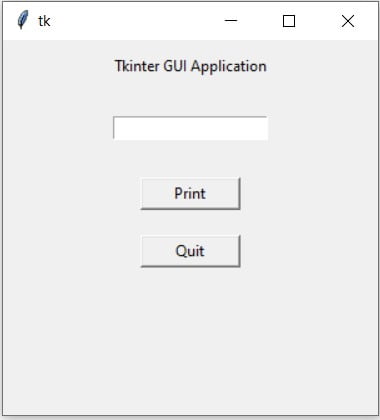
PyQt5
from PyQt5.QtWidgets import *
import sys
def display():
print(line_edit.text())
def quit_window():
window.close()
app = QApplication(sys.argv)
window = QMainWindow()
window.setGeometry(400,400,300,300)
window.setWindowTitle("CodersLegacy")
label = QLabel(window)
label.setText("PyQt5 GUI Application")
label.adjustSize()
label.move(90, 30)
line_edit = QLineEdit(window)
line_edit.move(100, 70)
button = QPushButton(window)
button.setText("Print")
button.clicked.connect(display)
button.move(100, 130)
button2 = QPushButton(window)
button2.setText("Quit")
button2.clicked.connect(quit_window)
button2.move(100, 170)
window.show()
sys.exit(app.exec_())
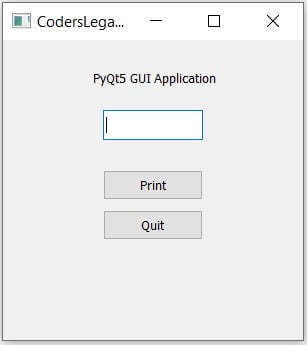
Another interesting thing to note is that the size of a PyQt5 compiled program is much larger than that of Tkinter. This probably has something to do with the fact that Tkinter is part of the Python Standard Library. You can try using an EXE converter like pyinstaller to test this out.
Update: PyQt6 has released since January 2021. You can find out more about this new version and its changes here.
Licensing
There is one last factor that might influence your decision slightly. Unlike Tkinter, PyQt5 was released under the GPL License. The GPL License forbids a person from distributing software using PyQt without including the source code.
Now obviously if you’re developing a commercial application, you will want to keep the source code with yourself. What you have to do then is to buy a commercial license for Qt that costs around $500.
For most use cases this doesn’t matter, and if you’re an actual company of group of developers, $500 for a permanent license isn’t a big deal. It is a factor in the PyQt vs Tkinter debate though, so we mentioned it.
If you really want to avoid this whole licensing issue, you can use the alternative to PyQt5 called PySide2.
Conclusion
So which one is the best GUI library? If I had to pick, it would be PyQt. Tkinter is good, and more than suitable for simple tasks, but PyQt just offers more overall in terms of widgets and looks.
If you’ve already learnt Tkinter and are comfortable with it, switching over isn’t really compulsory, just a recommended thing to do somewhere down the line. But if you haven’t begun with either Tkinter or PyQt, I strongly recommend you go ahead with PyQt.
Hopefully the comparisons and descriptions we showed you today have helped you decide the answer to the PyQt vs Tkinter question.
This marks the end of the PyQt vs Tkinter article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.