Welcome to the wxPython Tutorial Series. In this Tutorial Series we will cover the entirety of wxPython, from it’s basic setup all the way to advanced widgets and special features.
About wxPython
wxPython is a GUI library based of the popular C++ wxWidgets library. It’s a popular GUI library used by many for it’s cross-platform ability, clean UI and powerful and easy to use widgets.
Other popular GUI libraries similar to wxPython are Tkinter and PyQt.
wxPython Tutorial Series
In this tutorial (found below) we will begin by explaining how to setup a basic wxPython window and the various settings that come with it. The other widgets and other features are covered in other separate tutorials to which links can be found below.
A List of Tutorials on Widgets in wxPython:
- Basic Setup + Frames + Panels
- Button Widget
- StaticText Widget
- TextCtlr Widget
- StaticLine Widget
- RadioButton Widget
- RadioBox Widget
- ComboBox Widget
- CheckBox Widget
- ToggleButton Widget
- StatusBar Widget
- MenuBar and MenuButton Widget
- BitMapButton Widget
- StaticBox Widget
Once you have learnt how to create a few basic widgets, you should begin with these tutorials. These will teach you how to better manage and customize your wxPython application.
- Managing Widget Layouts using Sizers
- Events and Event Handling
- Colour with wxColour
- Making a ToolBar in wxPython
Downloading wxPython
The first thing you need to do is download wxPython using pip
, or some other package installer for your OS.
pip install wxpython
Next you just have to make the proper import in your Python code.
import wx
If this works without any errors, then your download/installation was successful.
Tutorial: Creating a wxPython Window (Frame)
In this section we’ll fully setup a proper Window using wxPython.
The below code implements a basic implementation of a wx.Frame,
which is used to create the Window. (We are using the object oriented approach here, which is flexible and effective in larger programs).
Within this frame is Panel widget, which is basically your “content window”, where you will be placing all of your widgets. You can create multiple panels as well, basically creating several areas where you can place widgets. The panel
has several parameters, but we will only pass one for the parent, which is the frame. (self refers to the frame
in this context)
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
self.panel = wx.Panel(self)
self.Show()
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
The __init__()
function takes an extra parameter for the title, otherwise the other parameter options for the wx.Frame
are left to their default values. Since this window is our main Window, the parent
is set to None
.
self.Show()
is a method belonging to the Frame Class, which is responsible for displaying the Frame on screen. There will be no output without this line.
Running the above code will produce the following output.
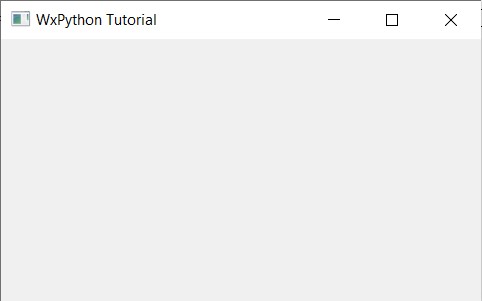
A full list of parameters that the wx.Frame
can take.
frame = wx.Frame(parent, id, title, pos,
size, style)
parent
: This is the Widget to which it is parented, such as a Panel.id
: Widget ID. Default value is ID_ANY, which gives it the next available ID.title:
The caption/name that appears on the topleft corner of the wxPython Window.pos:
A tuple containing the screen coordinates of where the topleft corner of the Window should begin from.size:
A tuple which defines the dimensions of the area occupied by the Window.style:
Used for styling the Window.
Styling the Window
In this section, we’ll take a look at some Styling options, which will add extra functionality into our Window.
A list of available Window Styles. The first style in this list is the default style that is already present whenever you create a Frame. It is actually a combination of many other styles inside this list. Hence, if you change the style from the Default to some other style, you will lose all of those default styles. (In that case you need to combine together various styles).
Window Style | Description |
---|---|
wx.DEFAULT_FRAME_STYLE | A compilation of several styles, wx.MINIMIZE_BOX | wx.MAXIMIZE_BOX | wx.RESIZE_BORDER | wx.SYSTEM_MENU | wx.CAPTION |wx.CLOSE_BOX | wx.CLIP_CHILDREN |
wx.ICONIZE | Display the Frame minimized (Windows only) |
wx.CAPTION | Puts a Caption Bar on the Frame. You must have this Style on for wx.MINIMIZE_BOX , wx.MAXIMIZE_BOX and wx.CLOSE_BOX to work properly on most systems. |
wx.MINIMIZE | Display the Frame minimized (Windows only) |
wx.MINIMIZE_BOX | Enables the minimize option on the Window. |
wx.MAXIMIZE | Display the Frame maximized (Windows and GTK Only) |
wx.MAXIMIZE_BOX | Enables the maximize option on the Window. |
wx.CLOSE_BOX | Enables the close option on the Window. |
wx.STAY_ON_TOP | Makes the Window stay on top of all other windows. |
wx.SYSTEM_MENU | Displays a system menu containing the list of various windows commands in the window title bar. |
wx.RESIZE_BORDER | Allows for resizing along the borders of the window. |
Using Window Styles in wxPython
Here is small example showing you how to use a Window Style on a Frame in wxPython.
frame = wx.Frame(parent = None, title = "Example", style = wx.MINIMIZE)
Refer to the table above to understand what this style does.
You can also pass in several styles by using the | (pipe) operator as shown below.
frame = wx.Frame(parent = None, title = "Example", style = wx.MINIMIZE | wx.CLOSE_BOX | wx.RESIZE_BORDER )
wx.Frame Methods
A list of useful methods that can be used on the Frame widget.
Method | Description |
---|---|
Show() | Displays the Frame on-screen. |
Centre() | Centers the Frame to the Screen. |
SetSize(x, y) | Used to change the size of the size of the Frame. |
Close() | Closes the Frame safely. |
Destroy() | Destroys the Frame immediately. |
Disable() | Disables the Frame. |
Hide() | Hides the window. |
Check out the documentation for the full list of available methods (if needed).
Other GUI Frameworks in Python
wxPython is one of many awesome GUI frameworks in Python. If you are interested in learning about the others, you can read this article on “The best GUI frameworks in Python“. Or if you want to directly compare wxPython to other competitors, here is a great resource for that.
This marks the end of the wxPython GUI Tutorial Series. Any suggestions or Contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.